Golang provides built-in data types like integer, string, float, etc which is very useful for writing simple programs but if you want to write a complex program, you need to create your data types. Struct in Golang helps you to define your data types. If you depend on Golang built-in data types, your code will be very complex and readability is low. Struct gives you the flexibility to create your data types.
- Define a new Struct in Golang
- Create or Initialize an Instance of a struct data type
- Create or Initialize an Instance of a struct data type using the new keyword
- Access Fields of a struct
- Embedded Struct or Types
- References
Define a new Struct in Golang
A struct is a type that contains named fields.
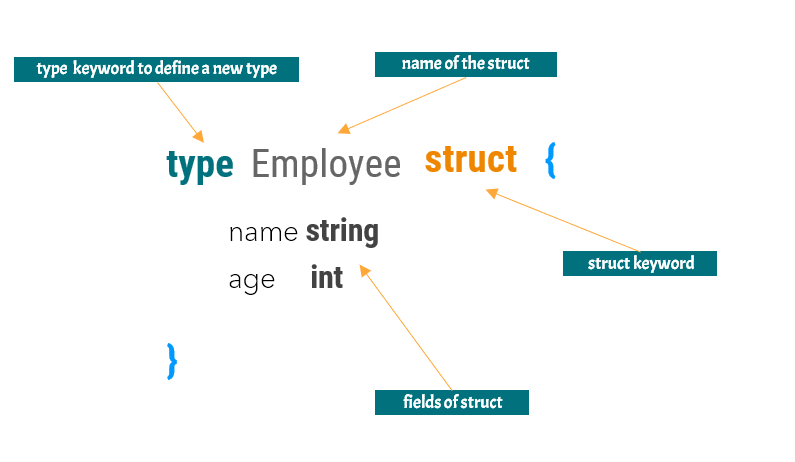
Defining a struct has 4 parts
- type keyword: Define a new type in Golang. You can define a struct or an interface etc, using the type keyword
- Name of Struct: You need to give a unique name to the struct. Here we have given the name as Employee
- struct keyword: It is used to create a new type of struct
- fields: You can add any number of fields to the struct. These are the properties of your new data type.
Let’s say you want to create an Employee management system then you will create an Employee Data type and give meaningful information or properties to the Employee data type.
type Employee struct {
firstName string
middleName string
lastName string
email string
age int
gender string
active bool
address string
}
In the above code, we have defined a new data type named Employee. You can create different instances of Employee and use them in your code
Create or Initialize an Instance of a struct data type
There are different ways to initialize a struct data type. The most common way is to give the fields an initial value while initializing the struct.
employeeOne := Employee{
firstName: "Raj",
lastName: "Ranjan",
email: "selftuts@gmail.com",
address: "Bengaluru India",
}
we have created the employeeOne instance from the Employee struct. You can see that when initializing the struct it is not mandatory to provide value to every field. If the value is not provided then a default value is automatically assigned.
Create or Initialize an Instance of a struct data type using the new keyword
You can use the new Keyword of Golang to create a new instance of the struct
employeeOne := new(Employee)
- The fields are assigned default values
- 0 for integer and float data type
- empty for string data type
- This allocates memory for all the fields, sets each to its default value, and returns a pointer to the struct (*Employee).
Access Fields of a struct
You can use the dot notation to access the fields of a struct instance
package main
import "fmt"
type Employee struct {
firstName string
middleName string
lastName string
email string
age int
gender string
active bool
address string
}
func main() {
employeeOne := Employee{
firstName: "Raj",
lastName: "Ranjan",
email: "selftuts@gmail.com",
address: "Bengaluru India",
}
// using the dot notation to access the field of a struct instance
fmt.Println(employeeOne.firstName)
fmt.Println(employeeOne.lastName)
fmt.Println(employeeOne.email)
}
➜ go run main.go
Raj
Ranjan
selftuts@gmail.com
Embedded Struct or Types
If your data is complex or nested, you will embed one struct inside another. This is called Embedded Struct or Embedded Types. The address is identified using multiple properties like city, flat number, state, and country. If you want to create a better data model then you will first create an Address struct and then use the Address struct inside the Employee struct
// first we define the Address struct
type Address struct {
flatNumber string
city string
state string
country string
}
type Employee struct {
firstName string
middleName string
lastName string
email string
age int
gender string
active bool
Address string // use Address struct as Embedded Type in Employee struct
}
You can see in the above Employee struct that the Address struct is an Embedded type.