An array in Golang is a numbered sequence of elements of the same type with a fixed length.
- Array Representation
- Declare an Array in Golang
- Declare and Initialize an array simultaneously in Golang
- Index of an Array in Golang
- Read or Access the value of an Array
- Insert or Write values to an Array in Golang
- Length of an Array in Golang
- References
Array Representation
An array can be treated as a box having multiple slots in it. Each slot can store the same type of item inside it

In the above picture, you can see that
- The Size of an array is fixed. Once it is defined then it can’t be modified
- The slots are storing only integer type of data. There is no mix of integer and string and boolean
Declare an Array in Golang
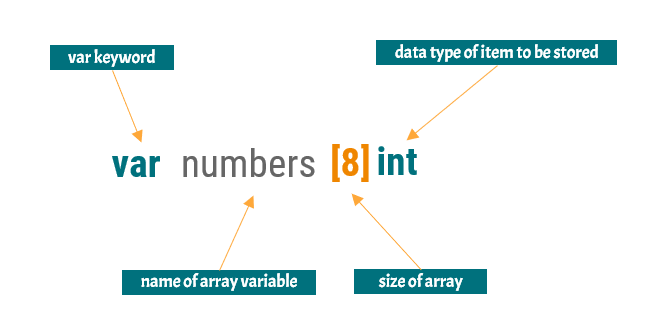
Golang provides the array keyword to declare a variable of array type. There are four parts to declaration of an array
- Use the var keyword
- Give a name to the array
- Define the size of the array
- Give the data type of the item to be stored inside the array. This can be
- integer
- string
- boolean
- another struct type
Once you declare the array then automatically assign the default value to each slot based on the data type of the array
- The default value of an integer is 0
- The default value for a string in an empty string “”
- The default value for float is 0
In our case, we have defined an integer array type so the default value in all the slots will be 0
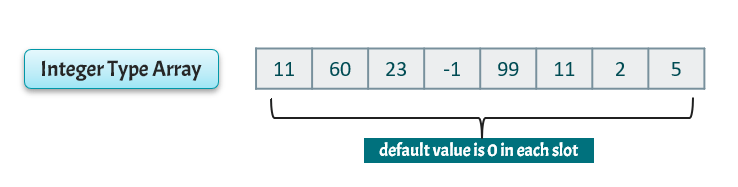
Declare and Initialize an array simultaneously in Golang
You can declare and initialize an array at the same time using curly bracket notation
var numbers = [8]int { 11, 60, 23, -1, 99, 11, 2 , 5 }
Index of an Array in Golang
Each slot of an array is associated with a particular index number. This index number is used to perform two different operation
- Accessing the value of a particular index
- Setting the value of a particular index
Index value always starts from 0 and not from 1 and the maximum value is one less than the size of the array
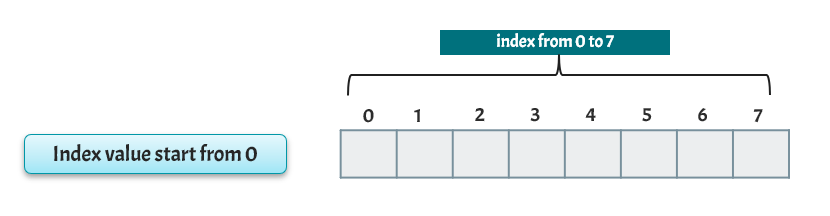
Read or Access the value of an Array
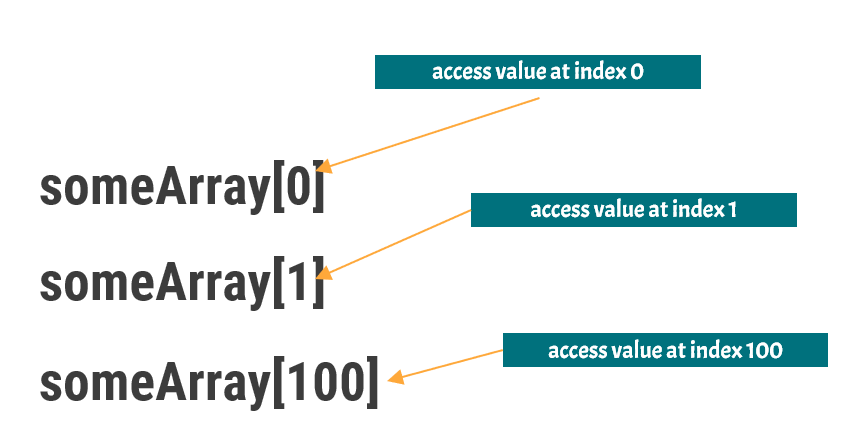
You can use the bracket notation to access the value at a particular index of an array. Let’s create an integer array of size 6 and access the value of each index
package main
import "fmt"
func main() {
var numbers = [6]int{11, 60, 23, -1, 99, 11}
fmt.Println("Value at index 0: ", numbers[0])
fmt.Println("Value at index 1: ", numbers[1])
fmt.Println("Value at index 2: ", numbers[2])
fmt.Println("Value at index 3: ", numbers[3])
fmt.Println("Value at index 4: ", numbers[4])
fmt.Println("Value at index 5: ", numbers[5])
}
➜ go run main.go
Value at index 0: 11
Value at index 1: 60
Value at index 2: 23
Value at index 3: -1
Value at index 4: 99
Value at index 5: 11
Insert or Write values to an Array in Golang
You can use bracket notation to write a value at a particular index of an array. Let’s create an integer array of size 6 and then add values.
package main
import "fmt"
func main() {
var numbers [6]int
// add values to the array
numbers[0] = 1
numbers[1] = 2
numbers[2] = 3
numbers[3] = 4
numbers[4] = 5
numbers[5] = 6
// read values from the array
fmt.Println("Value at index 0: ", numbers[0])
fmt.Println("Value at index 1: ", numbers[1])
fmt.Println("Value at index 2: ", numbers[2])
fmt.Println("Value at index 3: ", numbers[3])
fmt.Println("Value at index 4: ", numbers[4])
fmt.Println("Value at index 5: ", numbers[5])
}
➜ go run main.go
Value at index 0: 1
Value at index 1: 2
Value at index 2: 3
Value at index 3: 4
Value at index 4: 5
Value at index 5: 6
Length of an Array in Golang
The total number of elements in an array is the length of the array. You can find the length of an array using the len() function
len(arr)
package main
import "fmt"
func main() {
var numbers [6]int
numbers[0] = 1
numbers[1] = 2
numbers[2] = 3
numbers[3] = 4
numbers[4] = 5
numbers[5] = 6
// calcuate the length of array using
size := len(numbers)
fmt.Println(size)
}