Constants in Golang are essentially variables whose values can’t be changed later on.
- Declare and Initialize the Constant in Golang
- Rules for naming the Constant in Golang
- Declare and Initialize multiple constants in Golang simultaneously
- Write a program to create a constant and then change the value
- References
Declare and Initialize the Constant in Golang
We have seen that we can independently initialize Go variables. But for Go constants, this is not possible you need to both declare and initialize at the same time otherwise it will throw an error
The const keyword helps to create a constant in Golang. There are four parts to it
- use the const keyword
- give a name to the constant
- assign the data type either integer or string etc
- Finally, assign the value to the constant
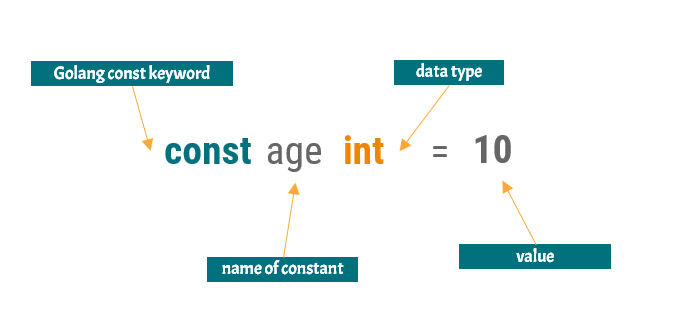
const age int = 10
Rules for naming the Constant in Golang
- The name must start with a letter
- It may contain underscore, letters, and numbers
- Go Compiler doesn’t care about the name of your variable but it should clearly describe the purpose of your variable
Declare and Initialize multiple constants in Golang simultaneously
You can use a shorthand to declare and initialize multiple constants at once. You need to use the const keyword followed by parenthesis with each constant on its line
Define and print three integer constants at once
package main
import "fmt"
func main() {
// define three integers constant at one time
const(
age = 10
count = 100
frequency = 50
)
fmt.Println(age)
fmt.Println(count)
fmt.Println(frequency)
}
Bash
➜ go run main.go
10
100
50
Define and print three string constants at once
package main
import "fmt"
func main() {
const (
name = "Code with Raj Ranjan"
country = "India"
city = "Bengaluru"
)
fmt.Println(name)
fmt.Println(country)
fmt.Println(city)
}
Bash
➜ go run main.go
Code with Raj Ranjan
India
Bengaluru
Write a program to create a constant and then change the value
package main
import "fmt"
func main() {
const a int = 100
// trying to reassign the constant
a = 200
fmt.Println(a)
}
Bash
➜ go run main.go
# command-line-arguments
./main.go:7:2: cannot assign to a (neither addressable nor a map index expression)
- Output Analysis
- The program returns an error which is expected because you cannot reassign a constant value