For loop in Golang allows to repeat a list of statements multiple times. Other programming languages have different kinds of loops like while, do, and until but Go has only one loop which is the for loop
- Components of a For Loop in Golang
- Write a program to print numbers from 1 to 10
- Write a program to print all even numbers from 1 to 100
- References
Components of a For Loop in Golang
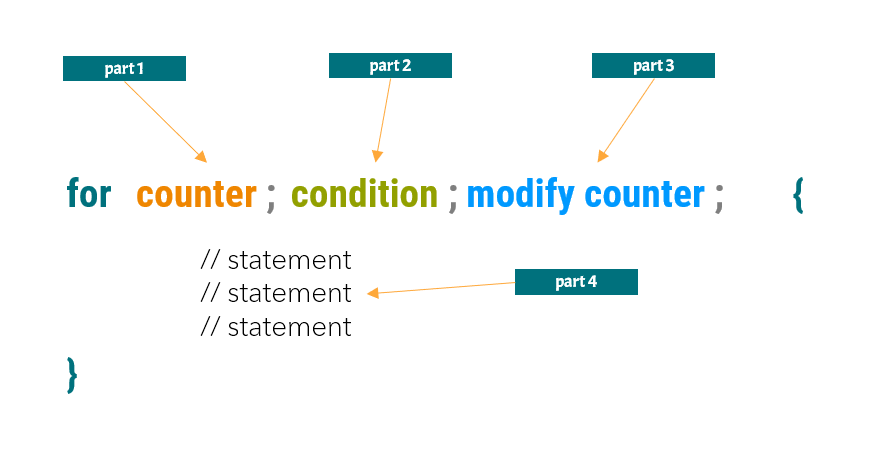
A for loop has four parts and each part is separated with a semicolon
- counter
- This is the start point from where the loop will start
- You can assign it any value like 0, 1, or -1
- condition
- Every time the loop starts running then it will evaluate the condition
- if the condition is true then the control will go inside the loop
- if the condition is false then the loop will not run and control will go to the lines below the loop
- You can take one of the condition examples as a counter is less than 100
- Every time the loop starts running then it will evaluate the condition
- Statements
- These are actual codes that you want to run when control enters inside your loop.
- You can write any code logic with multiple statements
- modify counter
- Once the statement inside the loop is evaluated then you can modify the counter
- You can increment the counter based on your code logic or
- You can decrement the counter based on your code logic
- Once the statement inside the loop is evaluated then you can modify the counter
Write a program to print numbers from 1 to 10
You can do this program without using a for loop. As the range is small you can simply use the print statement 10 times.
package main
import "fmt"
func main() {
fmt.Println("1")
fmt.Println("2")
fmt.Println("3")
fmt.Println("4")
fmt.Println("5")
fmt.Println("6")
fmt.Println("7")
fmt.Println("8")
fmt.Println("9")
fmt.Println("10")
}
➜ go run main.go
1
2
3
4
5
6
7
8
9
10
Using the above program we achieved our results, But what if someone asks us to print numbers from 1 to 5000?
Are you going to write the print statement 5000 times? In this case, writing a print statement 5000 times doesn’t make sense. The best choice will be to use the for loop as we can print from 1 to 5000 in fewer lines of code.
Let’s write the original program to print numbers from 1 to 10 using a for loop. As we have told that a for loop has four parts so let’s break down the problem statement in that way
- counter
- The counter will start from 1 since we need to print numbers starting from 1
- condition
- As we need to print numbers up to 10, we should continue printing numbers that are less than or equal to 10
- Statements
- The statement will be to print the number
- modify counter
- As we need to print the numbers sequentially we will increment the counter by 1
package main
import "fmt"
func main() {
// stating the coutner with 1
// condition to print the number which are less than or equal to 10
// printing the counter
// incrmenting the counter by 1
for i := 1; i <= 10; i++ {
fmt.Println(i)
}
}
➜ go run main.go
1
2
3
4
5
6
7
8
9
10
You can see that using a for loop converted your ten lines of code to 3 lines. Whenever you need to repeat something then use a for loop in Golang
Write a program to print all even numbers from 1 to 100
All numbers that are divisible by 2 is called an even number. In Golang we check the divisibility by modulo operator (%). When you find the module or number1 with number2 and the value is zero then it means that number1 is completely divisible by number2
// this means that number1 is completely divisible by number2
number1%number2 == 0
For this program
- counter
- The counter will start from 1
- condition
- As we need to find all the even numbers up to 100 the condition will be to check all numbers less than or equal to 100
- Statements
- The statement will be to find the modulo of the number
- modify counter
- As we need to print the numbers sequentially we will increment the counter by 1
package main
import "fmt"
func main() {
for i := 1; i <= 100; i++ {
// check if number is divisible by 2
if i%2 == 0 {
fmt.Println(i)
}
}
}
➜ go run main.go
2
4
6
8
10
12
14
16
18
20
22
24
26
28
30
32
34
36
38
40
42
44
46
48
50
52
54
56
58
60
62
64
66
68
70
72
74
76
78
80
82
84
86
88
90
92
94
96
98
100
You can see that all the numbers printed are even numbers