Function in Golang is an isolated piece of statements that are assigned a unique name and can be used multiple times in your project.
- Advantages of using function
- Signature of a function in Golang
- Write a function that accepts two integer numbers and returns their sum
- Write to function to check if the given number is an even number or not
- Write a function that returns two values
- References
Advantages of using function
- It makes your code more readable.
- A long piece of code can be broken down into independent small functions and it increases code readability
- It makes your code more organized.
- It removes repetitive code from your application
- You define a functionality and give it a name of function. The same function can be used in multiple places and it removes repetitive code.
Signature of a function in Golang
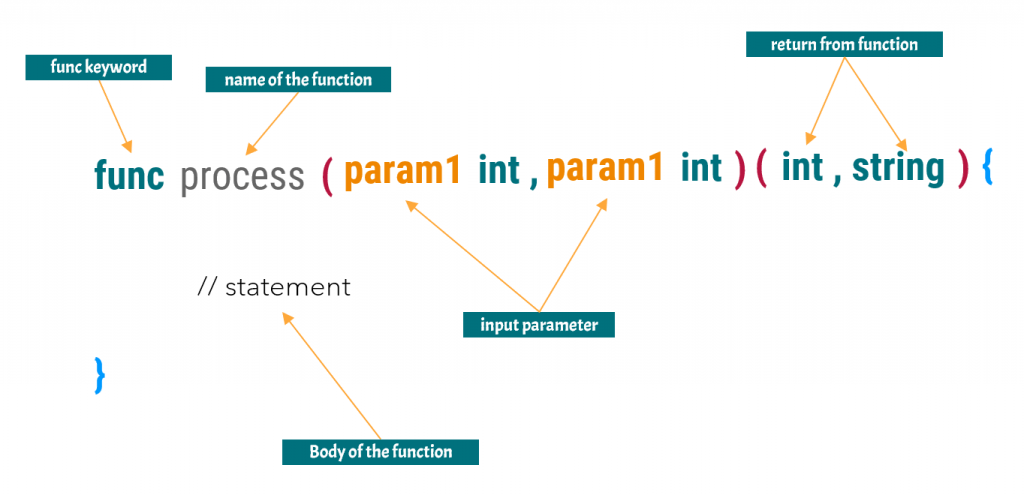
A function has five parts
- func keyword of Golang which tells the compiler, we are going to define a function
- Name of the function: Here process is the name of the function
- You can give any name to your function.
- Providing meaningful names to the function increases the code readability
- Input Parameters
- These are the inputs given to a function
- There is no restriction on the number of input parameter
- It can be zero
- It can be one or more than one
- When you define the input parameter then you need to give two things
- Name of the input parameter as it will be used with this name inside the function body
- The data type of the input parameter
- Return Values
- This defines what things can be returned from the function
- There is no restriction on the number of return values
- It can be zero
- It can be one or more than one
- You need to define the data type of the return values
- Body of the function
- These are the actual business logic statements that process input parameters and then return the values.
Write a function that accepts two integer numbers and returns their sum
Important point about this function
- The function accepts two parameters and the data type of both parameters is integer.
- As sum is returned the data type of the return value will also be an integer.
- We can define the name of the function as add
func add(number1 int, number2 int) (int) {
// returning the sum of two numbers
return number1 + number2
}
Write to function to check if the given number is an even number or not
In Golang we have remainder operator (%). If a number is completely divisible by another number then the remainder is zero
package main
import "fmt"
func main() {
var x int = 10
value := checkIsEven(x)
fmt.Println(value)
}
// function to check if the number is event or not
// accepts integer parameter
// returns bool value
func checkIsEven(n int) bool {
// using remainder operator to check if number is completely divisible by 2
if n%2 == 0 {
return true
}
return false
}
Bash
➜ go run main.go
true
Write a function that returns two values
As we know in Golang a function can return zero or one or more than one value. Let’s take a function that accepts an array of integers and returns the smallest and highest value of the array
package main
import (
"fmt"
"math"
)
func main() {
var numbers = []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
var lowest, highest = findHighestAndLowest(numbers)
fmt.Println("The highest number is", highest)
fmt.Println("The lowest number is", lowest)
}
func findHighestAndLowest(numbers []int) (lowest int, highest int) {
var lowestNumber int = math.MaxInt
var highestNumber int = math.MinInt
for _, number := range numbers {
if number < lowestNumber {
lowestNumber = number
}
if number > highestNumber {
highestNumber = number
}
}
return lowestNumber, highestNumber
}
Bash
➜ go run main.go
The highest number is 10
The lowest number is 1
- findHighestAndLowest Function explanation
- It accepts an integer array and returns two values the lowest and the highest number present in the array
- Using math, we predefined the lowest number as the highest value any integer variable can take math.MaxInt
- If the array contains any number then it will be lower than the highest value that an integer can take
- Using math, we predefined the highest number as the lowest value any integer variable can take math.MinInt
- If the array contains any number then it will be higher than the lowest value that an integer can take
- We check with every number that is present in the array and update the lowestNumber and highestNumber variable
- Finally, we return two values