An Integer in Golang are number comprising zero, positive numbers, and negative numbers. Integers like their match counterparts are numbers without decimal parts.
- Types of Integers
- When to use int and uint Integer data type
- Create an Unsigned Integer and print the value
- Create an Unsigned Integer and assign a negative value
- Create a signed Integer and print the value
- Create a signed Integer and assign a negative value
- Create 8 Bit Integer and assign a large value
- Write a program to add two integer numbers
- References
Types of Integers
There are different types of Go Integers
- uint8
- uint16
- uint32
- unit64
- int8
- int16
- int32
- int64
The numbers 8,16,32,64 denote the number of bits these types store. The more the number of bits the more larger number it will store. Every bit will be either 0 or 1
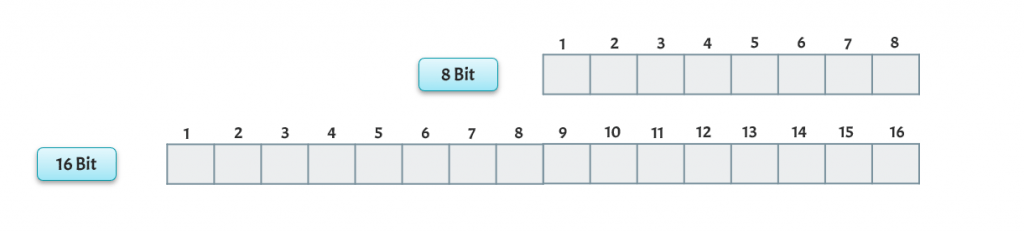
In the integer types, you must have noticed that bits are prefixed either with int or unit
- uint
- This denotes the unsigned int and can hold only positive integers including zero
- You cant store negative integers inside unsigned integers
- int
- This stores both negative and positive integers including zero
When to use int and uint Integer data type
If your program stores only positive numbers including zero and the numbers can be big then you can use the unit data type. For example
- The total number of views a YouTube video can get will always be zero or positive number. The count can reach up to billions, so using the uint64 data type is good.
Wherever you need to store both positive and negative values, you can use the non-signed integer data type. For example
- Profit and Loss in the stock market can be either a positive or negative number
Create an Unsigned Integer and print the value
package main
import "fmt"
func main() {
// x is an unsigned int
var x uint = 10
fmt.Println(x)
}
➜ go run main.go
10
This prints the value 10 on the screen
Create an Unsigned Integer and assign a negative value
package main
import "fmt"
func main() {
// assiging negative value to unsigned integer
var x uint = -10
fmt.Println(x)
}
➜ go run main.go
# command-line-arguments
./main.go:6:15: cannot use -10 (untyped int constant) as uint value in variable declaration (overflows)
The compiler gives an error cannot use -10 (untyped int constant) as uint value in the variable declaration (overflows)
Create a signed Integer and print the value
package main
import "fmt"
func main() {
// signed integer x which can hold both negative and positive value
var x int = 10
fmt.Println(x)
}
➜ go run main.go
10
Create a signed Integer and assign a negative value
package main
import "fmt"
func main() {
// assign negative value to a signed integer
var x int = -10
fmt.Println(x)
}
➜ hello-world go run main.go
-10
Create 8 Bit Integer and assign a large value
package main
import "fmt"
func main() {
// assign very large value to 8 bit integer
var x int = 100000000000000000000000
fmt.Println(x)
}
➜ go run main.go
# command-line-arguments
./main.go:6:14: cannot use 100000000000000000000000 (untyped int constant) as int value in variable declaration (overflows)
When the large value is assigned the compiler throws an error. You need to be vigilant in choosing the type of integer variable otherwise your application can crash during runtime.
Write a program to add two integer numbers
package main
import "fmt"
func main() {
var number1 int = 10
var number2 int = 20
fmt.Println(number1 + number2)
}
➜ go run main.go
30