if else in Golang is used to make some decisions. When the condition is satisfied then you can run some piece of code and when the condition is not satisfied then run another piece of code
- if Condition in Golang
- Else syntax in Golang
- if else if in Golang
- Write a program to check if a number is odd or even
- References
if Condition in Golang
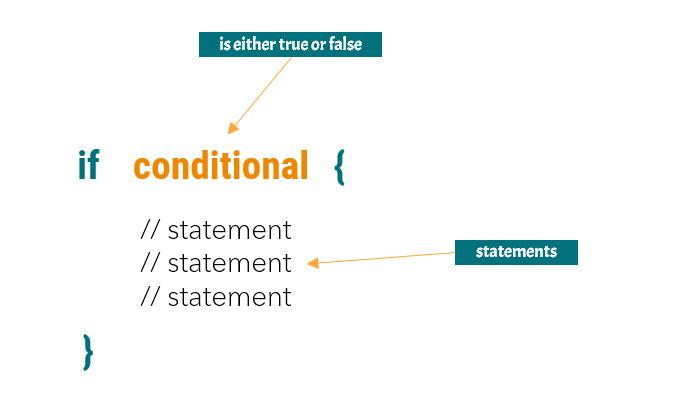
the if keyword helps you to write an if condition in Golang. The if condition consists of two parts
- Conditional Statement
- Here you write some expression that can be either true or false
- Statements
- when the condition is true then these statements are run
Let’s write a program where we make decisions on Age of a human. If the age is less than 10 years then we should print toddler
package main
import "fmt"
func main() {
var age int = 5
// the condtion is taken on age variable
// if the condtion is true then we will go inside the loop
if age < 10 {
fmt.Println("Toddler")
}
}
➜ go run main.go
Toddler
In the above program
- The age is 5 so the condition is satisfied and the control goes inside the if block and prints the statements
Now let’s say the age is 50 then the condition will not be satisfied and if the block is skipped
package main
import "fmt"
func main() {
var age int = 50
// as age is 50 so condition will not be satisfied
if age < 10 {
fmt.Println("Toddler")
}
}
➜ go run main.go
Else syntax in Golang
Every if condition has an optional else statement. It is not mandatory to write the else statement. Golang provides the else keyword for this.
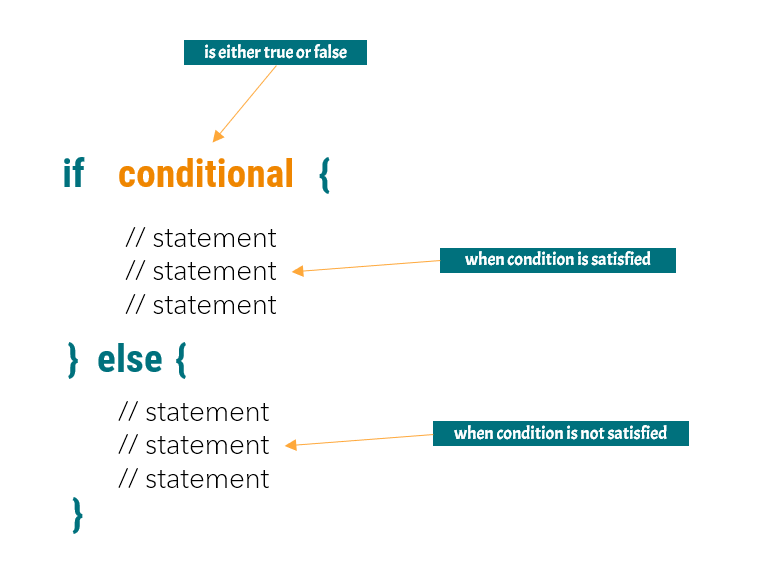
Program Control Flow
- First, the if condition is evaluated
- Condition is true
- Control goes inside the if block and executes the statement written inside if block
- Condition is false
- The program checks whether an else condition is present or not
- Else condition is present
- Then executes the statements inside the else block
- Else condition is not present
- Execution Control goes below the if block
- Else condition is present
- The program checks whether an else condition is present or not
- Condition is true
Let’s write a program where we make decisions on Age of a human. If the age is less than 10 years then we should print a toddler otherwise print not a toddler
package main
import "fmt"
func main() {
var age int = 50
// condition is not satisfied and the control goes to the else block
if age < 10 {
fmt.Println("Toddler")
} else {
fmt.Println("Not Toddler")
}
}
➜ go run main.go
Not Toddler
if else if in Golang
Golang provides the else if keyword to perform the chaining of conditions. Chaining of conditions can help you write complex code with more readability. There are two important points about chaining
- Evaluation happens from top to bottom
- When one condition is satisfied then others are not evaluated
- If none of the conditions is true then by default else block will run if it is present in the code
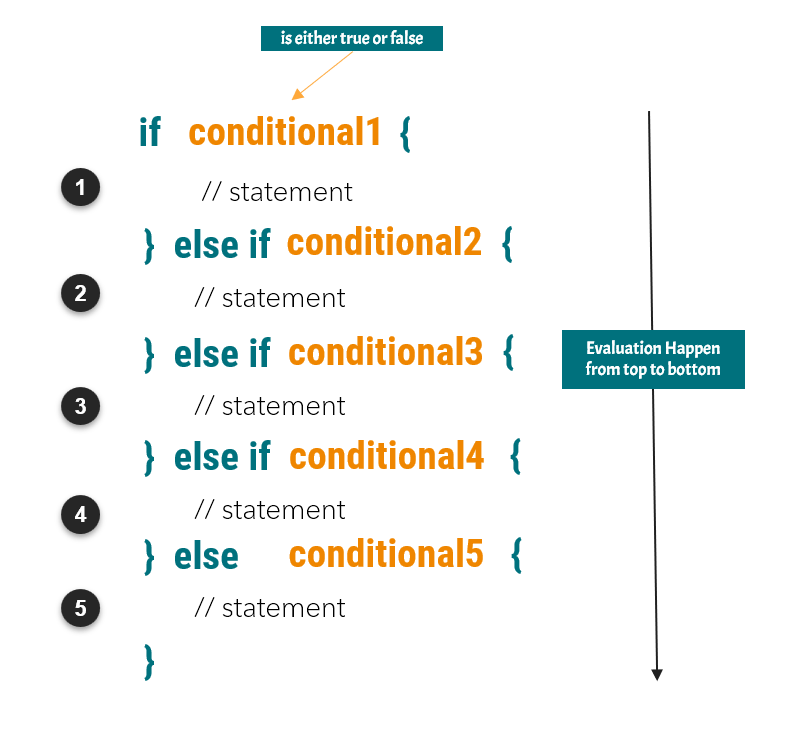
Important points to note from the above flow
- When Conditional1 is true then
- Statement Block 1 is only evaluated
- Statement Blocks 2, 3, 4, and 5 are skipped
- When Conditional2 is true then
- Statement Block 2 is only evaluated
- Statement Blocks 1, 3, 4, and 5 are skipped
- When Conditional3 is true then
- Statement Block 3 is only evaluated
- Statement Blocks 1, 2, 4, and 5 are skipped
- When Conditional4 is true then
- Statement Block 4 is only evaluated
- Statement Blocks 1, 2, 3, and 5 are skipped
- When none of the conditions are true then
- By default statement Block 5 is evaluated because it is else block
Now let’s extend our original program where we take the human age and apply the below logic
- If the age is less than 0 then print Invalid Age
- if the age is less than 10 then print the Toddler
- if the age is less than 20 but greater than or equal to 10 then print Boy
- if the age is less than 30 but greater than or equal to 20 then print Man
- if the age is less than 50 but greater than or equal to 30 then print the Old
- Else print Retired
package main
import "fmt"
func main() {
// you can change the value of age and then return the program
var age int = 10
if age < 0 {
fmt.Println("Invalid Age")
} else if age < 10 {
fmt.Println("Toddler")
} else if age >= 0 && age < 20 {
fmt.Println("Boy")
} else if age >= 20 && age < 30 {
fmt.Println("Man")
} else if age >= 30 && age < 50 {
fmt.Println("Old")
} else {
fmt.Println("Retired")
}
}
Write a program to check if a number is odd or even
A number is even if it is completely divisible by 2. Golang provides us modulo operator (%) to check the divisibility of one number to another
// if the value is zero then number1 is completely divisible by number2
number1%number == 0
package main
import "fmt"
func main() {
var number int = 20
// use modulo opertor to check divisibility
if number%2 == 0 {
fmt.Println("Number is even")
} else {
fmt.Println("Number is odd")
}
}
➜ go run main.go
Number is even