Map in Golang is an unordered collection of key-value pairs. A map is sometimes called an associative array, hash table, or dictionary
- Declare a Map in Golang
- Initialize a Map in Golang
- Read or Access the Key of a Map
- Insert or Write a key value to a Map in Golang
- Length or Size of a Map in Golang
- Delete an item from a map in Golang
- References
Declare a Map in Golang
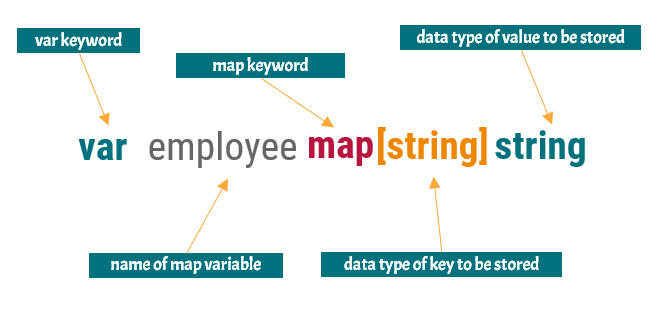
There are five parts to the declaration of an array
- Use the var keyword
- Give a name to the map
- Use the map keyword of Golang to define the map
- Define the data type of the key
- This defines the data type of the key to be stored. It can be anything integer, string, etc.
- Define the data type of the value
- This defines the data type of the value to be stored. It can be anything integer, string, or struct.
When you declare a map then it is just a nil map. If you perform some action on the declared map then it will throw an error. You can see the below program that throws an error
package main
import "fmt"
func main() {
// declared the map
var employee map[string]string
// trying to access only the declared map
employee["first"] = "John Doe"
fmt.Println(employee)
}
➜ go run main.go
panic: assignment to entry in nil map
goroutine 1 [running]:
main.main()
/home/selftuts/workspace/go/hello-world/main.go:8 +0x2c
exit status 2
Initialize a Map in Golang
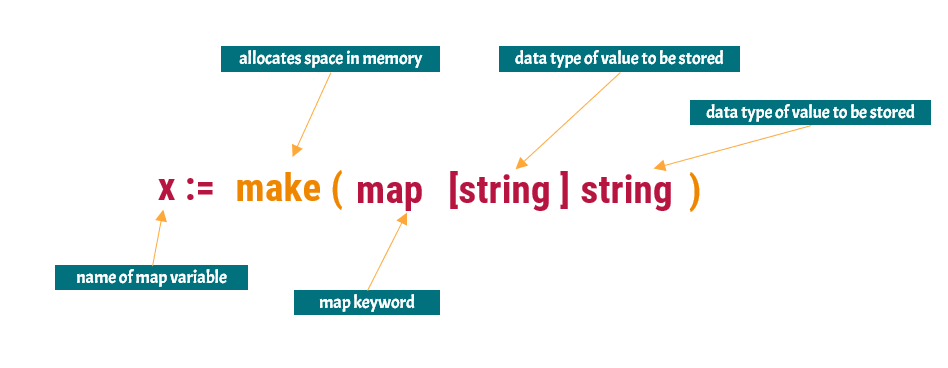
There are five parts to declare and initialize a slice in Golang
- Define the name of the map
- Use the make keyword to allocate some space in the memory which can be used to store the items
- Use the map keyword of Golang to tell that we are going to create a map
- The data type of the key to be stored in the map using the bracket notation
- The data type of the value to be stored in the map
Read or Access the Key of a Map
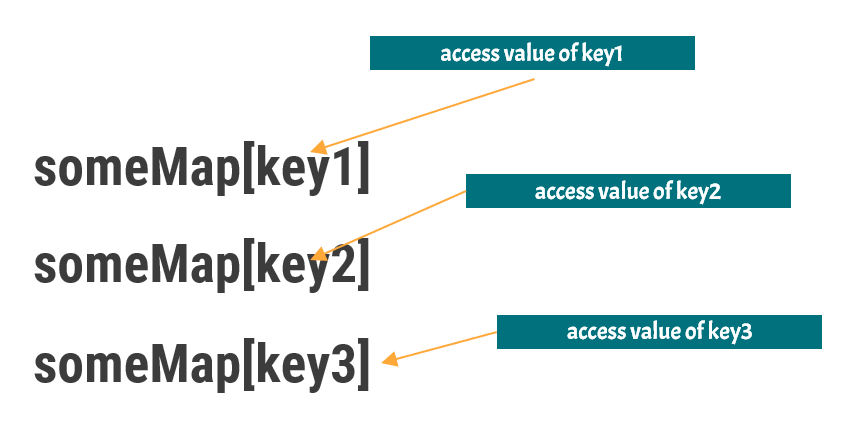
You can use the bracket notation to access the value of a particular key of a Map. Let’s create a map of programming language and its founder. When you access the key in the map then it returns two values first value is the result of the lookup and the second value is whether the lookup is successful or not
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
value, ok := languageFounder["rust"]
// access the key of a map
fmt.println(ok)
fmt.Println(value)
}
You can see that if the key is present then the value of ok is true. This can help you to decide that the key is present on the map.
➜ go run main.go
true
Graydon Hoare
If the key is not present then the value of ok is false
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
// access the key which is not present
value, ok := languageFounder["ruby"]
fmt.Println(ok)
fmt.Println(value)
}
➜ go run main.go
false
Insert or Write a key value to a Map in Golang
You can use the bracket notation to write key values in a Map.
- If the key is not present then it will add a new key to the map and the value will be inserted against the key
- If the key is already present then it will replace the value which is already present
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
}
Let’s try to add me as a founder for Go Language. This will overwrite the existing value of Rob Pike and add my name to the Map
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
// add my name as the value agaist the key go
languageFounder["go"] = "Raj Ranjan"
fmt.Println(languageFounder["go"])
}
It will print my name in the output
➜ go run main.go
Raj Ranjan
Length or Size of a Map in Golang
You can use the len function to find the size of a map in Golang. It returns the total number of keys present on the map
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
fmt.Println("The length of map is", len(languageFounder))
}
➜ go run main.go
The length of map is 6
Delete an item from a map in Golang
You can use the delete method to delete a key from the map. It accepts two arguments
- The map from which the item is to be deleted
- The key that is to be deleted
package main
import "fmt"
func main() {
var languageFounder map[string]string
languageFounder = make(map[string]string)
languageFounder["java"] = "James Gosling"
languageFounder["python"] = "Guido van Rossum"
languageFounder["c"] = "Dennis Ritche"
languageFounder["c++"] = "Bjarne Stroustrup"
languageFounder["go"] = "Rob Pike"
languageFounder["rust"] = "Graydon Hoare"
fmt.Println("The size of the map is", len(languageFounder))
delete(languageFounder, "c++")
fmt.Println("The size of the map is", len(languageFounder))
}
➜ go run main.go
The size of the map is 6
The size of the map is 5
After deletion, the size of the map becomes 5