- Exception classes in Java
- User Create Controller
- User Management Server Create User RPC
- Start the server
- References
Let’s learn to create a user in our user management service. User can register in the service by providing their details like first name, last name, email address, password, etc, and then use the email address and password to log into the system to generate a JWT Access Token.
- Part 1: What we are going to Build | User Management Service | Java | GRPC
- Part 2: MySQL Database | User Management Service | Java GRPC
- Part 3: GRPC Server Java | User Management Service
- Part 4: Create User in User Management Service GRPC Java
- Part 5: JWT token Java | User Management Service Java GRPC
- Part 6: Login API with JWT | User Management service Java GRPC
Exception classes in Java
we are following the software development paradigm where inner classes will throw exceptions in case of any error and then the controller level will catch the exception and then return a meaningful error response to the user. Let’s define a few exception classes that we will use
Create the exception directory and create two files Exception4XX and Exception5XX
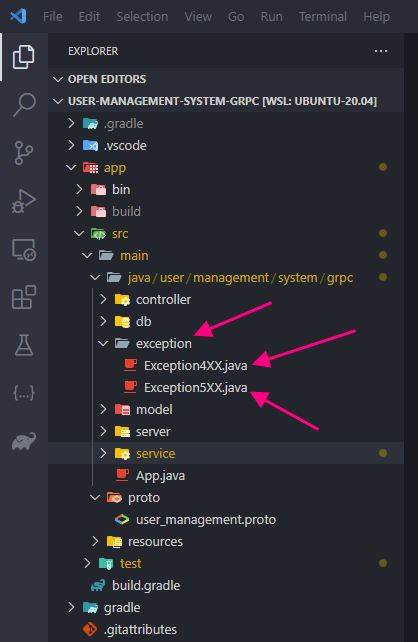
User-defined Exception4XX class
This exception will be raised when some known errors occur and user input is the reason for causing the error. For example, if the user provided the wrong email address and we need to raise an Exception then we throw this exception.
package user.management.system.grpc.exception;
public class Exception4XX extends Exception {
private String code;
private String entity;
private String message;
public Exception4XX(String code, String entity, String message) {
super(message);
this.code = code;
this.entity = entity;
this.message = message;
}
public String getCode() {
return code;
}
public String getEntity() {
return entity;
}
public String getMessage() {
return message;
}
}
- Inheritance
- Exception Class
- For any class to behave as an Exception class you need to inherit from the Exception Class of Java
- Exception Class
- Variables
- code
- These are specific codes that you can use to uniquely identify the errors. like 10000 for user not found etc. It depends fully on your choice to assign code values
- entity
- This is the item that caused the error. For example email_address
- message
- The detailed error message which helps you to understand the reason for the error.
- code
- Public Methods
- Getters for the variables have been defined
User-defined Exception5XX class
This exception will be raised when some unknown errors occur and user input is not the reason for causing the error. For example, connection with the database breaks and we need to raise an Exception then we throw this exception.
package user.management.system.grpc.exception;
public class Exception5XX extends Exception {
private String code;
private String entity;
private String message;
public Exception5XX(String code, String entity, String message) {
super(message);
this.code = code;
this.entity = entity;
this.message = message;
}
public String getCode() {
return code;
}
public String getEntity() {
return entity;
}
public String getMessage() {
return message;
}
}
User Create Controller
The controller plays an important role that doing all the heavy work in any application. It is the orchestration place that talks with multiple entities of your application. The User Create Controller will help orchestrate the user creation process.
- Validation of fields
- Inserting the data into MySQL database
- Return response to the client
Create the controller folder and create UserCreateController.java class
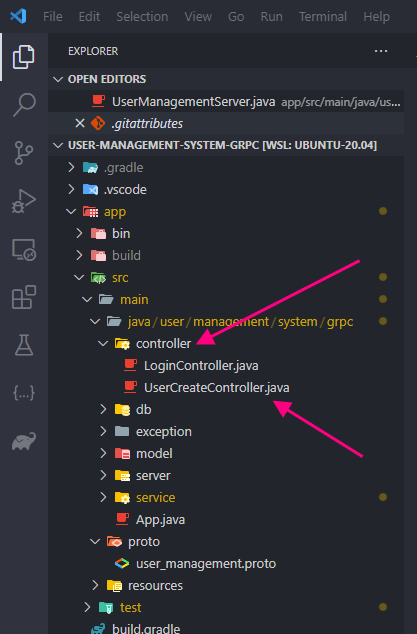
package user.management.system.grpc.controller;
import java.sql.SQLException;
import com.cwrr.user_management.CreateUserRequest;
import user.management.system.grpc.db.UserRepository;
import user.management.system.grpc.exception.Exception4XX;
import user.management.system.grpc.exception.Exception5XX;
import user.management.system.grpc.model.User;
public class UserCreateController {
UserRepository userRepository;
public UserCreateController(UserRepository userRepository) {
this.userRepository = userRepository;
}
public int createUser(CreateUserRequest createUserRequest) throws Exception4XX, Exception5XX, SQLException {
if(createUserRequest.getFirstName().isEmpty()) {
throw new Exception4XX("300", "first_name", "First Name can not be empty");
}
if(createUserRequest.getEmail().isEmpty()) {
throw new Exception4XX("300", "email", "Email can not be empty");
}
if(createUserRequest.getPassword().isEmpty()) {
throw new Exception4XX("300", "password", "Password can not be empty");
}
User user = new User();
user.setFirstName(createUserRequest.getFirstName());
user.setLastName(createUserRequest.getLastName());
user.setEmail(createUserRequest.getEmail());
user.setPassword(createUserRequest.getPassword());
if(userRepository.findUserByEmail(createUserRequest.getEmail()) != null) {
throw new Exception4XX("301", "email", "user already exists with this email");
}
userRepository.CreateUser(user);
User createdUser = userRepository.findUserByEmail(user.getEmail());
return createdUser.getId();
}
}
- Instance Variables
- userRepository
- This is the userRepository database instance.
- It is responsible for performing operations on the user database table
- UserRepository.java class was created in the previous post
- userRepository
- Public Methods
- createUser
- Accept the CreateUserRequest and return the ID of the newly created user.
- In case of errors throw exceptions like Exception4XX, Exception5XX
- createUser
- Code Logic
- Validation of incoming fields is performed
- Validation Fails
- Return error to user
- Validation Success
- Check if the user with an email exists in the database
- User Already Exists
- Return Error
- User Not Exists in the database
- Create a User in the database
- Return success response
- User Already Exists
- Check if the user with an email exists in the database
- Validation Fails
- Validation of incoming fields is performed
User Management Server Create User RPC
we have already added the createUser RPC in the UserManagementServer.java file. Now let’s add the logic where we integrate it with the UserCreateController
Add the below content for your createUserRPC
package user.management.system.grpc.server;
import com.cwrr.user_management.CreateUserResponse;
import com.cwrr.user_management.Error;
import com.cwrr.user_management.UserManagementServiceGrpc.UserManagementServiceImplBase;
import user.management.system.grpc.controller.UserCreateController;
import user.management.system.grpc.db.Database;
import user.management.system.grpc.db.UserRepository;
import user.management.system.grpc.exception.Exception4XX;
import user.management.system.grpc.exception.Exception5XX;
public class UserManagementServer extends UserManagementServiceImplBase{
UserCreateController userCreateController;
public UserManagementServer() {
Database db = new Database();
UserRepository userRepository = new UserRepository(db);
userCreateController = new UserCreateController(userRepository);
}
@Override
public void createUser(com.cwrr.user_management.CreateUserRequest createUserRequest, io.grpc.stub.StreamObserver<com.cwrr.user_management.CreateUserResponse> responseObserver) {
try {
int userId = userCreateController.createUser(createUserRequest);
CreateUserResponse createUserResponse = CreateUserResponse.newBuilder()
.setSuccess(true)
.setUserId(userId)
.build();
responseObserver.onNext(createUserResponse);
responseObserver.onCompleted();
} catch(Exception5XX e){
e.printStackTrace();
Error error = Error.newBuilder().setCode(e.getCode()).setEntity(e.getEntity()).setMessage(e.getMessage()).build();
CreateUserResponse createUserResponse = CreateUserResponse.newBuilder()
.setSuccess(false)
.setError(error)
.build();
responseObserver.onNext(createUserResponse);
responseObserver.onCompleted();
} catch(Exception4XX e){
e.printStackTrace();
Error error = Error.newBuilder().setCode(e.getCode()).setEntity(e.getEntity()).setMessage(e.getMessage()).build();
CreateUserResponse createUserResponse = CreateUserResponse.newBuilder()
.setSuccess(false)
.setError(error)
.build();
responseObserver.onNext(createUserResponse);
responseObserver.onCompleted();
} catch (Exception e) {
e.printStackTrace();
Error error = Error.newBuilder().setCode("900").setEntity("internal server error").setMessage("internal server error").build();
CreateUserResponse createUserResponse = CreateUserResponse.newBuilder()
.setSuccess(false)
.setError(error)
.build();
responseObserver.onNext(createUserResponse);
responseObserver.onCompleted();
}
}
@Override
public void login(com.cwrr.user_management.LoginRequest loginRequest, io.grpc.stub.StreamObserver<com.cwrr.user_management.LoginResponse> responseObserver) {
}
}
- Instance Variables
- userCreateController
- This controller holds the orchestration for creating the user
- userCreateController
- Public Methods
- UserManagementServer Constructor
- we initialize all the important dependencies that are needed by the server to start
- createUser
- This is the method where the request will reach first when someone calls createUser RPC
- This calls the createUser method present in UserController
- All the Exceptions are handled and a meaningful response is returned to the user.
- UserManagementServer Constructor
Start the server
You can start the server and use any GRPC client like the Postman to hit this RPC and start creating users
./gradlew run

This was all about the user creation
Previous: Part 3: GRPC Server Java | User Management Service
Next: Part 5: JWT token Java | User Management Service Java GRPC