- Public and Private Keys for JWT token
- Java Dependency for JWT token generation
- Public and Private Key Generator/Supplier for JWT Library
- Java JWT token generator
- Sample Token
- References
In today’s world of microservice deployment, architecture is widely used and if you want to perform authentication and authorization across multiple services then JSON Web Token (JWT) is widely used. we will also create JWT when a user does a successful authentication. Let’s learn to create the JWT token in our User Management service.
- Part 1: What we are going to Build | User Management Service | Java | GRPC
- Part 2: MySQL Database | User Management Service | Java GRPC
- Part 3: GRPC Server Java | User Management Service
- Part 4: Create User in User Management Service GRPC Java
- Part 5: JWT token Java | User Management Service Java GRPC
- Part 6: Login API with JWT | User Management service Java GRPC
Public and Private Keys for JWT token
For JWT token generation you need to choose an algorithm that will help you to sign your JWT token. Symmetric algorithms and Asymmetric algorithms are available like HMAC, and RSA256. In a symmetric algorithm, a single key is used to both create and verify the token. In an asymmetric algorithm, we have two keys one for signing the token and another for verifying the token.
- Private Key: This key is used for signing or creating the token
- Public Key: This key is used for verifying the token
we will be using the Asymmetric algorithm RSA256 in our user management service.
Generate Public and Private Keys
There are multiple ways to generate the private and public keys. we will be using the online website. visit the website for generating the keys
https://acte.ltd/utils/openssl
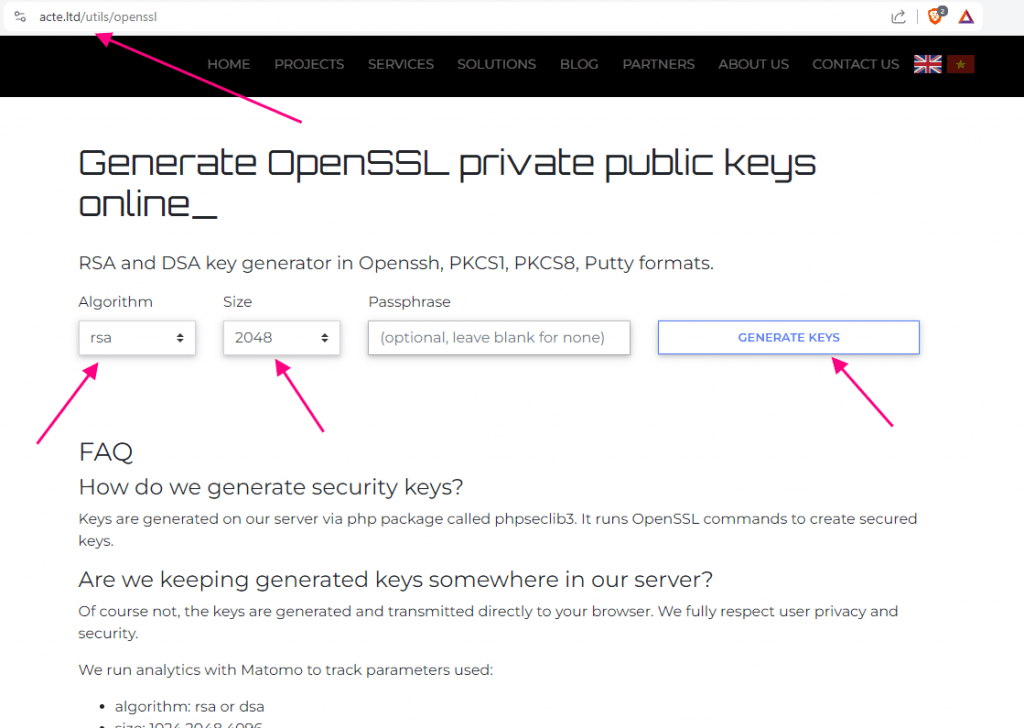
You need to select the options from the list and then click on Generate Keys
- Algorithm: RSA
- size: 2048
- Leave the passphrase empty
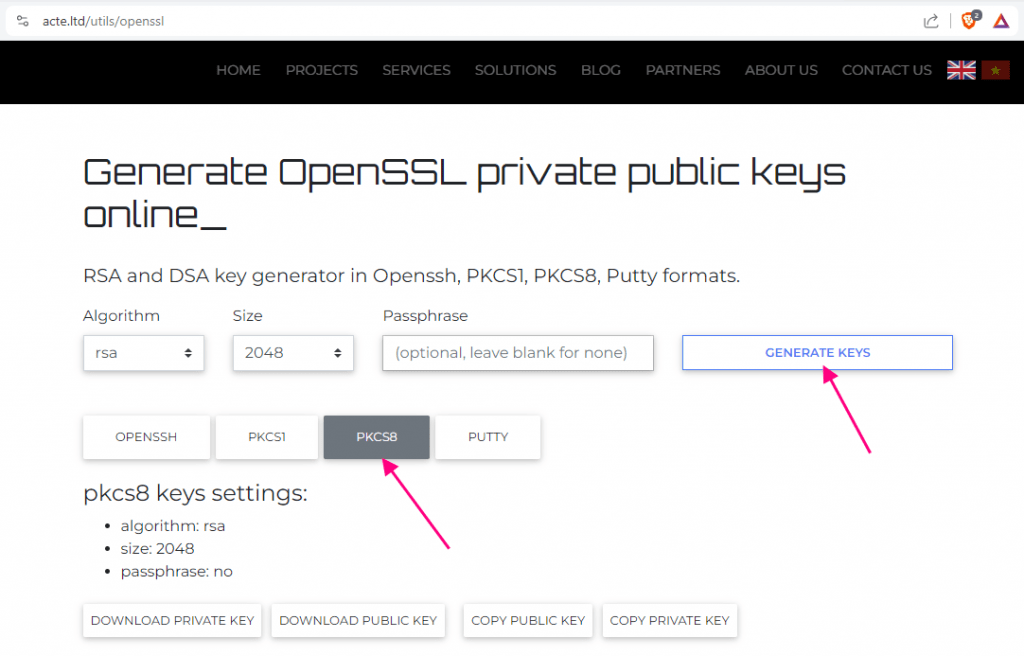
After clicking the GENERATE KEYS button click on the PKCS8 format. You will see the public and private keys at the bottom of the screen
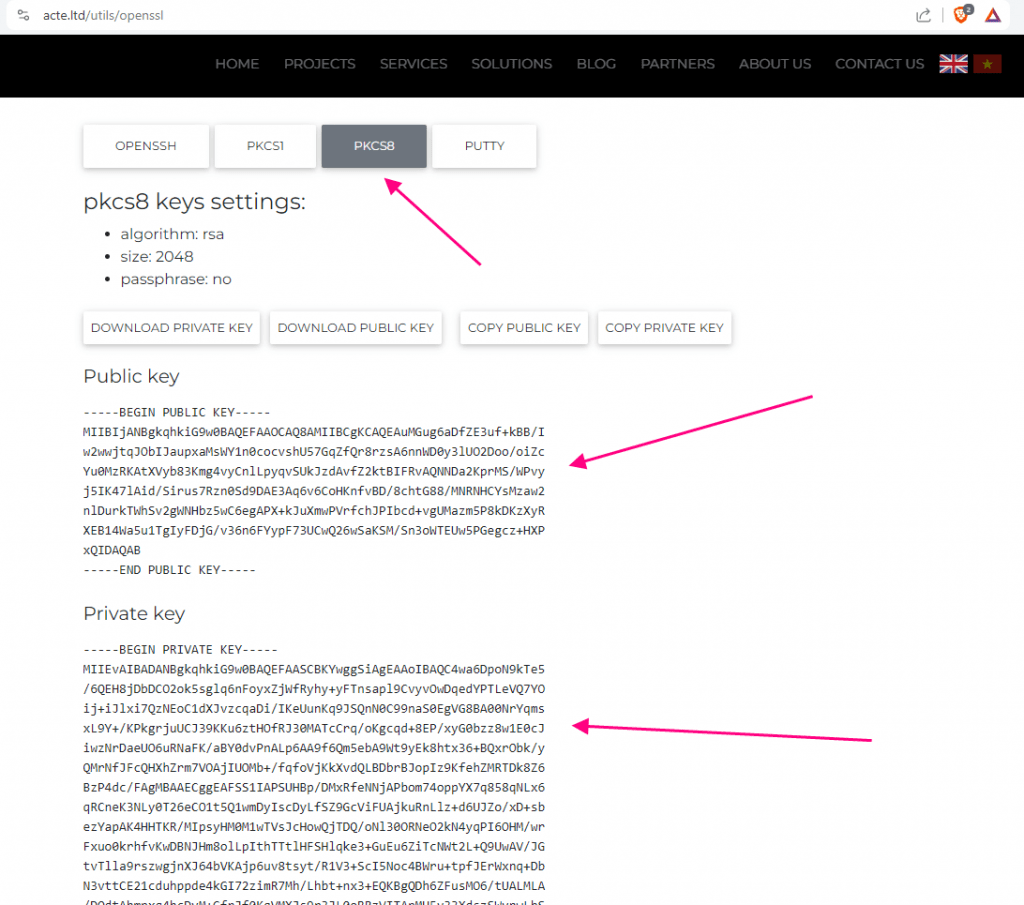
Store Public and Private keys in the Resources folder
Create the resources folder and then create two files
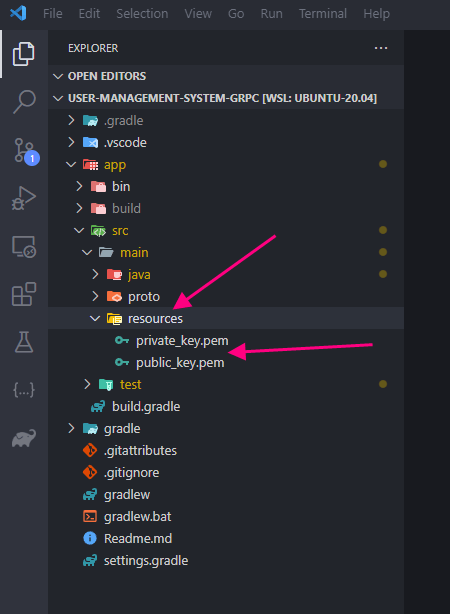
- private_key.pem
- public_key.pem
Copy the public key and private key from the website which we generated earlier and then save them in appropriate files.
The private key that we are using is
-----BEGIN PRIVATE KEY-----
MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQCgf5FzsDlpQmsn
mYFUgH5Zmie9i1XvbcPcXKo7RWS8dD6sGvSUA47I0CSi4Q+W+7hHnp9RnNxFWpNz
+/ZZ56bkCBSG0NMcc9j76AV9YkKbeK8DdSGhKji7ZaBxSv1atI6Qdnz2ascgjS6B
nloukHFRDUlpOOj66YXS7uHYNTXla2H69VYvgqA07GlAUKBA3LB7VDGa5irWIRlG
xI/73EsSBpv5T9mrGCFkTurOY27XDzm9bLwXZAwB59RAAwGcHjtDLSsTDLfEx04u
5t+woBbNZ71JRMCtHWmXPNr6i2958BdUFs6kTJTqlhZMZaFfmT2+5Sr7xRQS4tyy
w7xeCCE1AgMBAAECggEAFQRfgazD99qwuYDKhMxGv2kGZzXFzRKsoUCpMhXGOT9n
rZXiDTfiDL3k7kdWiUjxOr+00TUfSx2vTXLjMaa6HgZI2dairoklEuexQFgLrNxC
3ssJ2lbzEucd+Xf+cVBPku9RMduNs9+JBYQ8/vf/PsPkpxjCuYi284ZAoNVm92HB
Xezg6YXp2urMC3GD1lO1rFa6mrbxGRLMzJ6OHk5wV1UvG62psXeAvJ9WQ6FtB1lv
Z/WGM018v56FWhE7+CjC0eQ93DdySvPsYLdoKIBJg4533W8l2CjVfwrjSIiif71d
0Z3u62cDHVhM/rcXjrcejHgrMVEBAn2xh5FpOikOgQKBgQDMdjBS0qGH4EL9a9+4
aMmV8OdOrRJj94bfpbsEUngQ85sUR8HDHHPoXQBgEHvjFL01G5mXWk/2j9ivS2iI
7xSkgV3dIGmp/zrFT3KveaYAzpZ1Y961HqMnsdUBsaKMHvLtIL3QT91Oe8J3iU2V
xVhiAM8o77N/ZMwTtP1x8bk5hQKBgQDI9HIAXirLGzCkJDghiiwpJ+pDWxPqqjlm
sIIn00GLnvD5zAVd4GY67xUut7WICXsKnb17dY5D8nf/Sungnw4nSw4iNBq3UBKM
6SAKwEzkqVNbOpAB6Y7KZryOT0u2qLrG1nb8gAxIv74h7G9/ljxn5ULSksZHyy6W
uFy6mKB/8QKBgBMmNpxrMcnCDUQi6E8jMU4jw+Ywe3p1YmJr6TocZzZfOnUJy3H0
pj5rB2/320KHXLkunpH4WWjkTA0O6Pl6Otq1aw8czAip6N019a3NnOiScLEJ63/X
ILzFAQgplOm+tE3VNTHfaQDCwpHjgCq40vBK1xwkZxFVZW+rEdmsJAbpAoGAXwFy
hEoA4VVRgci94N16U1rTnlSI7gDccngwkLOH4YKbWv3h66gTrPkrgpLH+DDDQxmg
Y6YIRId1w/Se7dtEzb7mR7VqjBAHNSdiRrLzp4eGKTNesgjBwV8g9gb3PLw40ZjD
WE0RgbNlKJSngiJ9HIF/Ph62L2sX85nl/TH7b4ECgYEArZa0IdRvw/Zbu6aKc7SM
alPEC1LXsI4DZTjAuSyrE/A7I/UaBsqwjru1JWT0cFbsMWTI0+hhNgykqsD4mlGh
q97FB53jbol4rTkKRGskAiSn8fEiC/JMN+XbWv7AjMfe9Dv8mhfOl44LcQXvghoB
hmpYYLiidCu4798aiIYNRFA=
-----END PRIVATE KEY-----
The public key that we are using is
-----BEGIN PUBLIC KEY-----
MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAoH+Rc7A5aUJrJ5mBVIB+
WZonvYtV723D3FyqO0VkvHQ+rBr0lAOOyNAkouEPlvu4R56fUZzcRVqTc/v2Weem
5AgUhtDTHHPY++gFfWJCm3ivA3UhoSo4u2WgcUr9WrSOkHZ89mrHII0ugZ5aLpBx
UQ1JaTjo+umF0u7h2DU15Wth+vVWL4KgNOxpQFCgQNywe1QxmuYq1iEZRsSP+9xL
Egab+U/ZqxghZE7qzmNu1w85vWy8F2QMAefUQAMBnB47Qy0rEwy3xMdOLubfsKAW
zWe9SUTArR1plzza+otvefAXVBbOpEyU6pYWTGWhX5k9vuUq+8UUEuLcssO8Xggh
NQIDAQAB
-----END PUBLIC KEY-----
Java Dependency for JWT token generation
we need a library to generate the Java JWT token. we will be using auth0 java-jwt library for our project. let’s add the below library in the dependencies of your build.gradle file
implementation 'com.auth0:java-jwt:4.4.0'
Public and Private Key Generator/Supplier for JWT Library
we have downloaded the public and private keys and stored them in a file. Now we want to read the keys from the file and then supply it to the JWT library. Create a new folder service and add the JWTKeyGenerator.java file
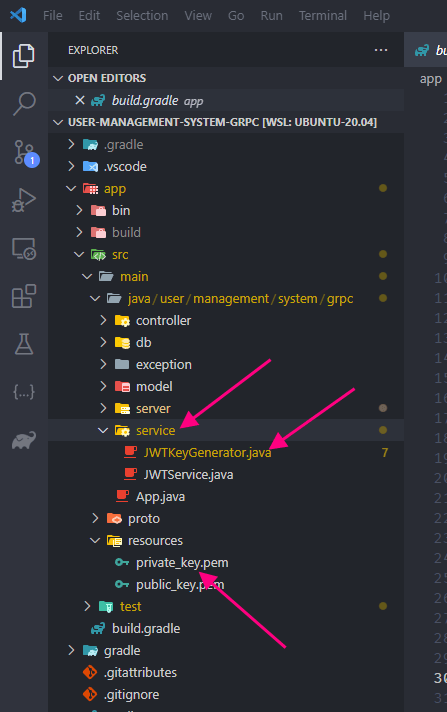
Put the below content in JWTKeyGenerator.java class
package user.management.system.grpc.service;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.KeyFactory;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Base64;
import user.management.system.grpc.exception.Exception5XX;
public class JWTKeyGenerator {
public RSAPublicKey getPublicKey() throws Exception5XX {
try {
String publicKeyContent = new String(Files.readAllBytes(Paths.get(ClassLoader.getSystemResource("public_key.pem").toURI())),Charset.defaultCharset());
publicKeyContent = publicKeyContent.replaceAll("\\n", "").replace("-----BEGIN PUBLIC KEY-----", "").replace("-----END PUBLIC KEY-----", "");
byte[] encoded = Base64.getDecoder().decode(publicKeyContent);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(encoded);
return (RSAPublicKey) keyFactory.generatePublic(keySpec);
} catch (Exception e) {
e.printStackTrace();
throw new Exception5XX("10000", "public key", "public key generation error");
}
}
public RSAPrivateKey getPrivateKey() throws Exception5XX {
try {
String privateKeyContent = new String(Files.readAllBytes(Paths.get(ClassLoader.getSystemResource("private_key.pem").toURI())),Charset.defaultCharset());
privateKeyContent = privateKeyContent.replaceAll("\\n", "").replace("-----BEGIN PRIVATE KEY-----", "").replace("-----END PRIVATE KEY-----", "");
privateKeyContent = privateKeyContent.replaceAll("\\s", "");
byte[] encoded = Base64.getDecoder().decode(privateKeyContent);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(encoded);
return (RSAPrivateKey) keyFactory.generatePrivate(keySpec);
} catch (Exception e) {
e.printStackTrace();
throw new Exception5XX("10000", "private key", "private key generation error");
}
}
}
- Public Methods
- getPublicKey
- Reads the public key from public_key.pem file of the resources folder and return the RSAPublicKey object
- getPrivateKey
- Reads the private key from private_key.pem file of the resources folder and return the RSAPrivateKey object
- getPublicKey
Java JWT token generator
Now we have the public and private key suppliers from the JWTKeyGenerator class. Let’s create the JWT token. Create a new file JWTService.java in the service folder
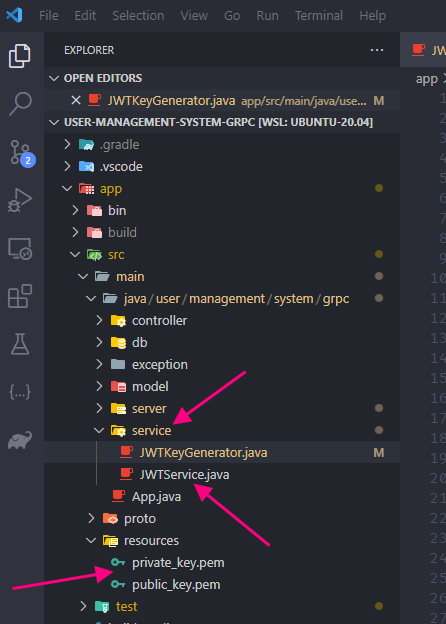
Put the below content in the JWTService.java
package user.management.system.grpc.service;
import java.util.Date;
import java.util.concurrent.TimeUnit;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import user.management.system.grpc.exception.Exception5XX;
public class JWTService {
JWTKeyGenerator jwtKeyGenerator = new JWTKeyGenerator();
public String generateToken(String firstName, String email, String lastName, int id) throws Exception5XX {
Algorithm algorithm = Algorithm.RSA256(jwtKeyGenerator.getPublicKey(), jwtKeyGenerator.getPrivateKey());
String token = JWT.create()
.withIssuer("user-managment-system-grpc")
.withClaim("firstName", firstName)
.withClaim("lastName", lastName)
.withClaim("email", email)
.withClaim("id", id)
.withExpiresAt(new Date(System.currentTimeMillis() + TimeUnit.MINUTES.toMillis(5)))
.sign(algorithm);
return token;
}
}
- Instance Variables
- jwtKeyGenerator
- This holds the instance of JWTKeyGenerator that is supplied through the constructor
- jwtKeyGenerator
- Public Methods
- generateToken
- Uses the RSA256 Algorithm and used the private and public key
- Claims are supplied which is nothing but key-value pair that will be present in the JWT token
- The token is signed with the algorithm and returned
- generateToken
Sample Token
The sample token which will be generated later on will be
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJ1c2VyLW1hbmFnbWVudC1zeXN0ZW0tZ3JwYyIsImZpcnN0TmFtZSI6InJhaiIsImxhc3ROYW1lIjoicmFuamFuIiwiZW1haWwiOiJhYmNAZ21haWwuY29tIiwiaWQiOjEyLCJleHAiOjE3MTQyODExNzd9.YJd9HFrg7k9FNzF-gMALVnI0e3eteGCFnTRE3E-OHyj4p1HfQasm46FyQZ4aXvmuxJDk-k9Viao9ZLvSRa73qENk2o6ewBBmjFrtYfA4ZIyfdiieCyvpxXcu4aEOpYOuBcXyrNm0Gq7_5erA5lglB0gbxBkUw9ig8IZmICA-JkcEGdPEVREyYv9bEu2lm0-_MC3IEbqCHBBNSy0Wz741O8vw4cYtLRKEjzHyWgl8LmrDNG-hdslqYmP8fQ8b5yF8pmeKzegfhI7i5dIZInCNJwNtq3n1egzRitVjl6txSRpdPFG8FqfGpbmZdJNfUfwfAAa2Q_bYJpbEnIiWGJjdkw
This is the process to generate a JWT token in Java
Previous: Part 4: Create User in User Management Service GRPC Java
Next: