Capturing the user input is a very important feature of any programming language. we can also read data or user input in Golang
- Scan Method of fmt package in Golang
- Read Multiple User Inputs using the Scan method in Golang
- References
Scan Method of fmt package in Golang
Golang provides an inbuilt package fmt which reads user input data. An inbuilt package comes within the language installation and there is no need to install anything separately.
The fmt package has a Scan method which reads the user input.
- When the program encounters a Scan method call then it stops the execution and waits for user input
- Once the input is given and the user presses Enter key on the keyboard then the control goes to the next line
- The value entered will be stored in the variable that is passed as a parameter to the Scan function
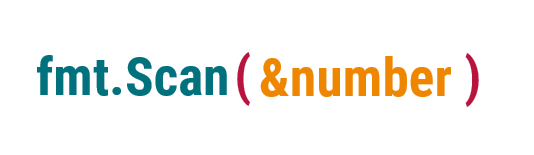
When the user presses enter then the value will be assigned to the number variable
Let’s create a program where we try to take a single user input
package main
import "fmt"
func main() {
// number variable where user input will be stored
var number string
// showing user a meanigul text when the program will wait for the user input
fmt.Println("Please Enter your number : ")
// using the Scan method on fmt package
fmt.Scan(&number)
fmt.Println("Your number is : ", number)
}
When you run this program the first thing the program will wait to take the user input
➜ hello-world go run main.go
Please Enter your number :
After you input a number and run hit Enter then further execution of the program will happen
➜ go run main.go
Please Enter your number :
55
Your number is : 55
If in the above program, you will provide multiple space-separated values then the Scan method will take only the first value and ignore others. You can see in the below output that only 66 is printed and then the next two numbers are ignored and passed to the terminal.
➜ hello-world go run main.go
Please Enter your number :
66 99 88
Your number is : 66
➜ 99 88
zsh: command not found: 99
Read Multiple User Inputs using the Scan method in Golang
If you want to read multiple inputs in the same line then you need to provide multiple variables inside the Scan function
Let’s say you want to read three variables from the user input.
package main
import "fmt"
func main() {
// three variable where user input will be stored
var number1 string
var number2 string
var number3 string
// showing user a meanigul text when the program will wait for the user input
fmt.Println("Please Enter your numbers : ")
// using the Scan method on fmt package to read multiple user input
fmt.Scan(&number1, &number2, &number3)
fmt.Println("Your first number is : ", number1)
fmt.Println("Your second number is : ", number2)
fmt.Println("Your third number is : ", number3)
}
➜ go run main.go
Please Enter your numbers :
12 14 14
Your first number is : 12
Your second number is : 14
Your third number is : 14
- Code Explanation
- You can see that we are taking three variables number1, number2, and number3
- In the Scan function, we are giving three variables to collect the three inputs provided by the user
- The user provides three inputs and then those are printed