Redis Pub Sub is an important feature supporting the publish-subscribe message paradigm. Let’s learn how we can use the ioredis npm module to create a publisher, subscriber, and channel using NodeJS
What we are going to build
we will create a publisher in NodeJS and start publishing messages from the publisher to a channel. Then we will create multiple subscribers of this channel, which will consume and print messages from the channel.
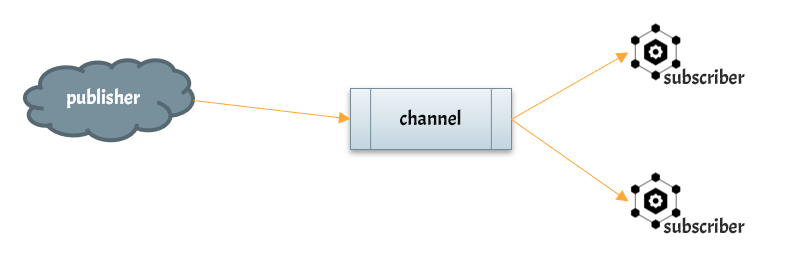
Prerequisite
- Redis must be installed
- You can follow Install Redis using docker-compose to set up your own Redis installation.
- NodeJS must be installed
Initialize the NodeJS project
Let’s set up a new NodeJS application. Navigate to the directory where you want to create your application
mkdir nodejs-redis-pub-sub
cd nodejs-redis-pub-sub
once you are inside the application directory then we will initialize the npm project
npm init
The NPM tool will ask you a few questions. Just keep hitting the enter/return key and this will create a package.json file in the project directory.
package.json file holds all the configuration related to your NodeJS project like dependencies, the main entry file, etc.
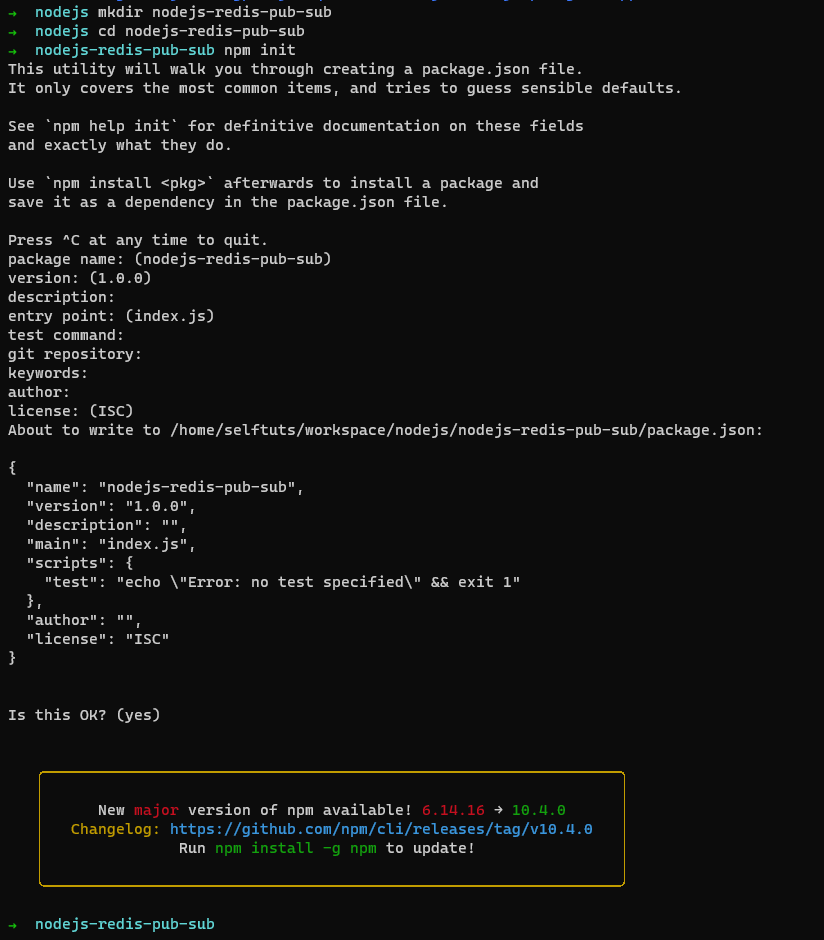
Install the ioredis npm module
ioredis is a robust, full-featured Redis client that is used in the world’s biggest online commerce company Alibaba, and many other awesome companies.
we will install the ioredis and save it as a dependency in the package.json file
npm install ioredis --save
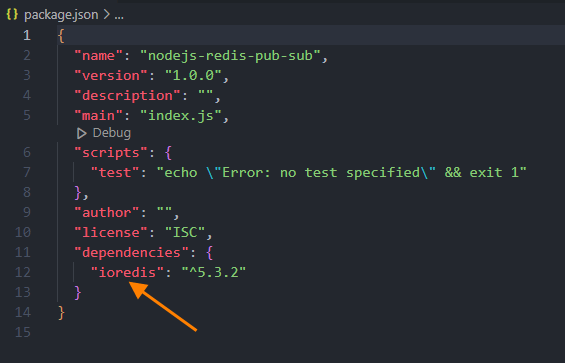
Connect to Redis
Follow the prerequisite section to install Redis.
Redis is installed in the local environment with
- host = localhost
- port = 6379
If you want to connect to the Redis instance then you need to provide some configuration for establishing the Redis connection
const config = {
port: 6379, // Redis port
host: "ocalhost", // Redis host
username: "default", // needs Redis >= 6
password: "my-top-secret"
}
In our case, we don’t have any authentication on the Redis server so we will not use the username and passport parameters
The code to connect to Redis will be
const redis = require("ioredis")
const config = {
"host": "localhost",
"port": 6379
}
const redisConn = new redis.Redis(config)
we imported the ioredis module and then created a new Redis connection using the config object.
Create Redis Publisher
Create a new file named publisher.js
touch publisher.js
const redis = require("ioredis")
const config = {
"host": "localhost",
"port": 6379
}
const redisConn = new redis.Redis(config)
redisConn.publish("task", "Hello, Redis!")
.then((result) => {
console.log("success", result)
}).catch((err) => {
console.log("error",err)
})
- Call the publish method on the redisConn and it accepts two parameter
- The first parameter is the channel name
- The second parameter is the message that you want to publish
- the publish method returns a promise object and you can handle the success and error scenario
- In the success, you get the result
- The value of the result will be the number of subscribers that are consuming from that channel
Once the publisher code is ready then we need to run the publisher.
node publihser.js
This will publish the message to the task channel because we have named the channel as task
Also if you want to publish the message multiple times then you need to run the publisher again and again.
Create Redis Subscriber
Create a new file named subscriber.js
touch subscriber.js
const redis = require("ioredis")
const config = {
"host": "localhost",
"port": 6379
}
const redisConn = new redis.Redis(config)
async function consume() {
let subscriber = await redisConn.subscribe("task")
redisConn.on("message", (channel, message) => {
console.log(`channel: ${channel}, message: ${message}`)
})
}
consume()
- You need to subscribe to a channel using the subscribe method
- It accepts one parameter and the parameter is the name of the channel
- we need to subscribe to the message event on redisConn that will invoke a callback when a new message is published to any channel
- The first parameter of the callback is the channel name
- The second parameter is the message
you need to run the subscriber such that it can start consuming messages from the task channel
node subscriber.js
Running the application
we will start with three consumers first and then run the publisher to publish the message.
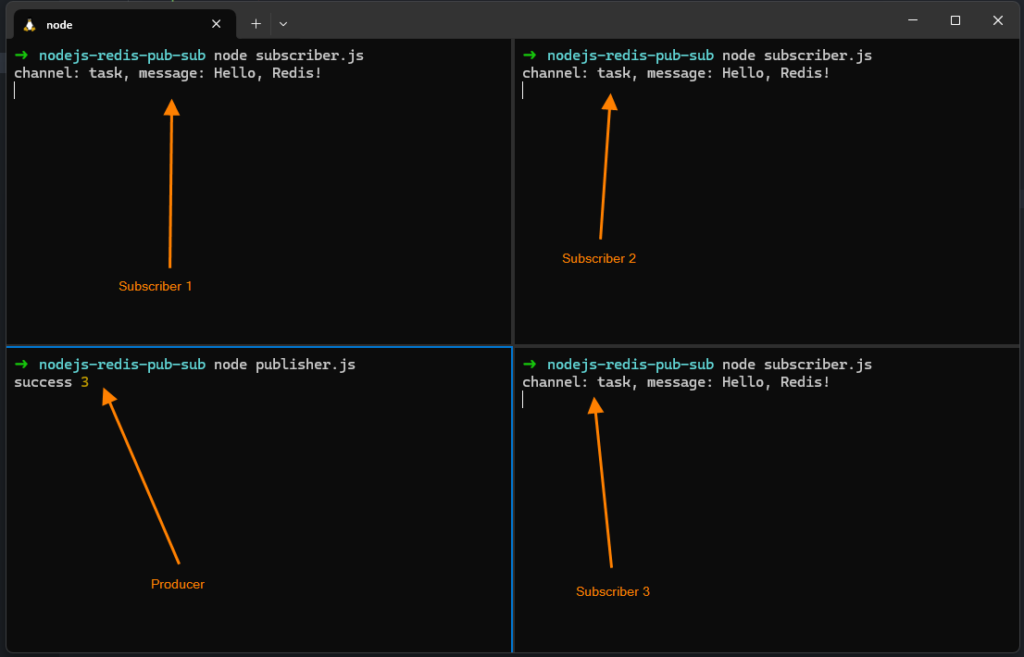
- You can see the message is written on all three consumer
- Also, you can see Success 3 printed in the publisher terminal
- Then number 3 signifies that three subscribers are attached to the channel