Slice is Golang is the segment of an array. Unlike an array, the length of a slice is allowed to change. The dynamic length of a slice is the most important feature of a slice like array slices are indexable and have length.
- Declaring a slice in Golang
- Length and Capacity of a slice
- Declaring and Initialize a slice with length and capacity
- Default Initialization of indexes till the length of a slice
- Create a Slice from an array in Golang
- Find the length and Capacity of a slice in Golang
- Read or Access the value of an Array
- Insert or Write values to a Slice in Golang
- Append Method on the slice
- Copy method on a Slice
- References
Declaring a slice in Golang
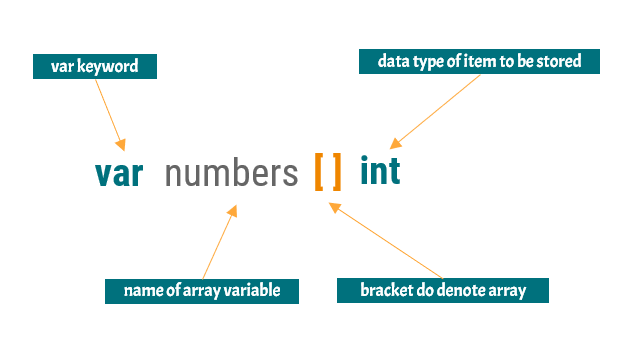
There are four parts to the declaration of an array
- Use the var keyword
- Give a name to the array
- Define the slice notation using brackets.
- Don’t provide any size in the bracket.
- Give the data type of the item to be stored inside the array. This can be
- integer
- string
- boolean
- another struct type
When you declare a slice then the length of the slice is zero. It is also called a nil slice. If you try to access any element of the slice at any index, it will throw an error. But this is not the same in an array, when you declare an array then it automatically initializes the array with the default value of the data type.
Length and Capacity of a slice
- The capacity of a slice refers to the total number of elements it can hold.
- The length of a slice refers to the number of elements it is currently holding
- when you provide the length of the slice then those number of elements will be automatically initialized with the default value of the data type
- The default value of an integer is 0
- The default value for a string in an empty string “”
- The default value for float is 0
- when you provide the length of the slice then those number of elements will be automatically initialized with the default value of the data type
Capacity can be greater than or equal to the length of the slice.
Declaring and Initialize a slice with length and capacity
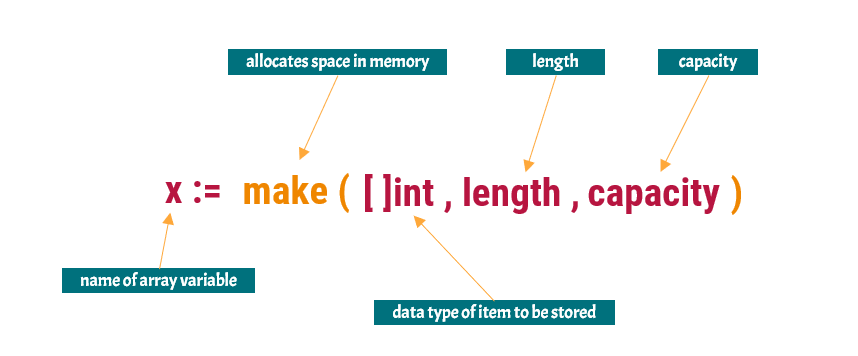
There are five parts to declare and initialize a slice in Golang
- Define the name of the slice
- Use the make keyword to allocate some space in the memory which can be used to store the items
- Length of the slice
- Capacity of the slice
Default Initialization of indexes till the length of a slice
Let’s say you create an integer slice with a length of 5 and capacity of 10 then the first five elements will be initialized with the default value of zero.
package main
import "fmt"
func main() {
// declare and initialize a slice
x := make([]int, 5, 10)
// access slice till the length
for i := 0; i < 5; i++ {
fmt.Println(x[i])
}
}
➜ go run main.go
0
0
0
0
0
Every time 0 is printed, the default value of the integer data type.
If you try to access till the capacity then Goland will throw an error
package main
import "fmt"
func main() {
// declare and initialize a slice
x := make([]int, 5, 10)
// access slice till the length
for i := 0; i < 10; i++ {
fmt.Println(x[i])
}
}
➜ go run main.go
0
0
0
0
0
panic: runtime error: index out of range [5] with length 5
goroutine 1 [running]:
main.main()
/home/selftuts/workspace/go/hello-world/main.go:9 +0xcb
exit status 2
You can see the output that up to index 4 zero is printed, but as soon as it reaches index 5, the sixth element, it throws an error.
Create a Slice from an array in Golang
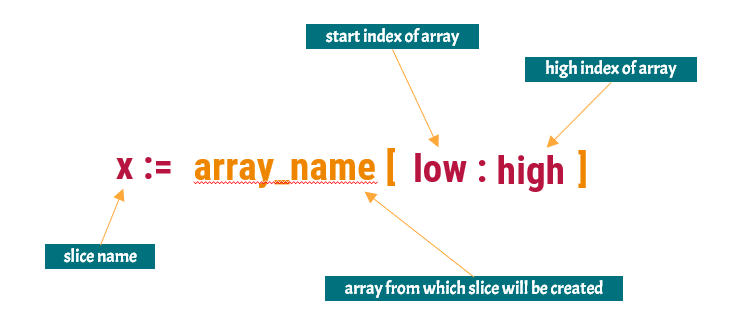
Another way to create a slice is by using the high and low expression on an array.
- You need to select the array from which the slice will be created
- Use the bracket notation
- Provide the low index from where elements will be picked
- Provide the high index up to which elements will be picked up
- Note: The high index is not included in the slice
- The high and low index are optional
You can create a slice between two indexes of an array. Below we are creating a slice from index 3 to index 6. The last index is not included so the elements in the slice will be 4, 5, 6
numbers:= [8]int{1,2,3,4,5,6,7,8}
x := numbers[3:6]
// note here the slice x will contain values 4,5,6
You can create a slice by only giving the low index. In this case, the slice will contain all the elements till the end of the array.
numbers:= [8]int{1,2,3,4,5,6,7,8}
x := numbers[3:]
// note here the slice x will contain values 4,5,6,7,8
You can create a slice without giving the high and low values. In this case, the slice will contain all the array elements.
numbers:= [8]int{1,2,3,4,5,6,7,8}
x := numbers[:]
// note here the slice x will contain values 1,2,3,4,5,6,7,8
Find the length and Capacity of a slice in Golang
The length is the actual number of elements present inside the slice. The length of a slice can be equal to less than the capacity of the slice.
- Golang provides the len method to find the length of the slice.
- Golang provides the cap method to find the capacity of the slice
package main
import "fmt"
func main() {
x := make([]int, 5, 10)
fmt.Println("Length of slice:", len(x))
fmt.Println("Capacity of slice:", cap(x))
}
➜ go run main.go
Length of slice: 5
Capacity of slice: 10
Read or Access the value of an Array
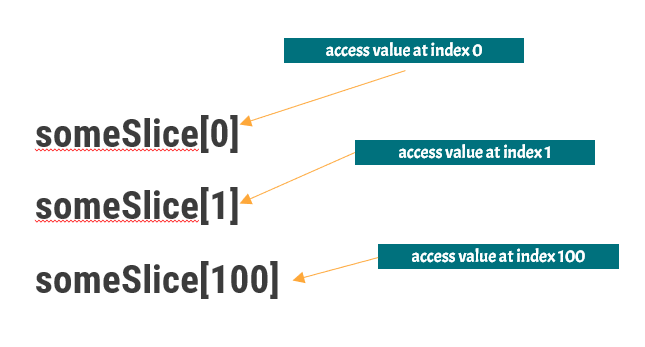
You can use the bracket notation to access the value at a particular index of a slice. Let’s create an integer slice and access the value of each index
package main
import "fmt"
func main() {
// creating a slice without giving the size
var numbers = []int{11, 60, 23, -1, 99, 11}
fmt.Println("Value at index 0: ", numbers[0])
fmt.Println("Value at index 1: ", numbers[1])
fmt.Println("Value at index 2: ", numbers[2])
fmt.Println("Value at index 3: ", numbers[3])
fmt.Println("Value at index 4: ", numbers[4])
fmt.Println("Value at index 5: ", numbers[5])
}
➜ go run main.go
Value at index 0: 11
Value at index 1: 60
Value at index 2: 23
Value at index 3: -1
Value at index 4: 99
Value at index 5: 11
Insert or Write values to a Slice in Golang
You can use bracket notation to write a value at a particular index of a slice. Let’s create an integer slice and add values.
package main
import "fmt"
func main() {
// create a slice
var numbers = make([]int, 6)
// add values to the slice
numbers[0] = 1
numbers[1] = 2
numbers[2] = 3
numbers[3] = 4
numbers[4] = 5
numbers[5] = 6
// read values from the slice
fmt.Println("Value at index 0: ", numbers[0])
fmt.Println("Value at index 1: ", numbers[1])
fmt.Println("Value at index 2: ", numbers[2])
fmt.Println("Value at index 3: ", numbers[3])
fmt.Println("Value at index 4: ", numbers[4])
fmt.Println("Value at index 5: ", numbers[5])
}
➜ go run main.go
Value at index 0: 1
Value at index 1: 2
Value at index 2: 3
Value at index 3: 4
Value at index 4: 5
Value at index 5: 6
Append Method on the slice
- The append method is used to add an element at the end of the slice
- It always adds the element at the end of the slice
- If there is capacity then add the element to the end and increase the length
- If there is no capacity
- A new slice is created,
- All of the existing elements are copied over and the new element is added to the end
- A new slice is returned
package main
import "fmt"
func main() {
// create a slice with two numbmers
var numbers = []int{1, 2}
fmt.Println(numbers)
// append 3 to the numbers slice
numbers = append(numbers, 3)
fmt.Println(numbers)
}
➜ go run main.go
[1 2]
[1 2 3]
- Output Explanation
- First, we create a slice with two elements 1 and 2
- We append element 3 to the slice and after printing, we see that element 3 is appended to the end of the slice
Let’s print the length and capacity after appending the element to the slice
package main
import "fmt"
func main() {
var numbers = make([]int, 3)
fmt.Println("The size of numbers is", len(numbers))
fmt.Println("The capacity of numbers is", cap(numbers))
numbers = append(numbers, 1)
numbers = append(numbers, 2)
numbers = append(numbers, 3)
fmt.Println(numbers)
fmt.Println("The size of numbers is", len(numbers))
fmt.Println("The capacity of numbers is", cap(numbers))
}
➜ go run main.go
[0 0 0]
The size of numbers is 3
The capacity of numbers is 3
[0 0 0 1 2 3]
The size of numbers is 6
The capacity of numbers is 6
Output Explanation
- When we created the slice then it was by default assigned values as zero as zero is the default value of an integer
- Then we appended elements 1, 2, and 3 to the slice and these were appended at the end of the slice even if the slice has default values.
- The length and capacity also increased after adding the elements
Copy method on a Slice
- Copy takes two slices and copies elements from one slice to another
- Two arguments are src and destination
- All the entries in src are copied to the destination overwriting whatever is there
- if the length of the two slices are not the same then the smaller of the two will be used