Switch in Golang helps you create different conditions and allows you to execute different codes when different conditions are evaluated.
- Switch with the case statement in Golang
- How control flow works in the Switch statement of Golang
- Write a program to return weekday from its integer values
- References
Switch with the case statement in Golang
Golang provides the switch keyword to create a switch construct and then you can different conditions based on the case keyword
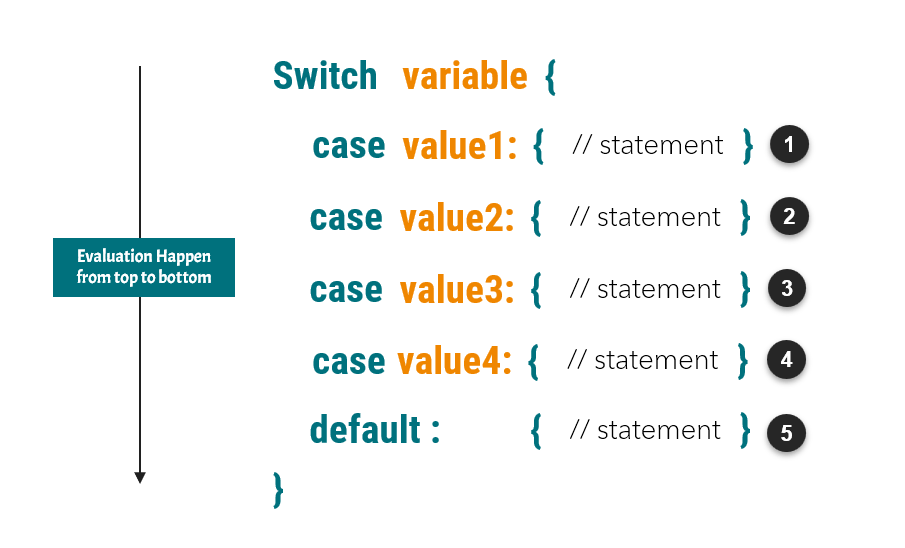
You will use a switch statement when you want to make different decisions based on the value of a variable.
Switch construct in Golang has three parts
- Select the Variable on which you want to make a decision using the switch keyword
- Define different flows for different values of the variable using the case keyword
- Default flow which will be invoked when none of the cases matches
- Default flow is optional. Based on your code logic you may or may not write the default case
How control flow works in the Switch statement of Golang
- The flow of evaluation is from top to bottom
- Duplicate cases are not allowed and the Golang compiler will throw an error
- If one of the cases is matched then only the statement of that case will run and other cases will be skipped
- The default case is optional.
- If the default case is present and none of the other cases are matched then only the default case will run
Let’s understand it using the above diagram
- When Case 1 is matched
- Statement of 1 will run
- Statements 2, 3, 4, and 5 are skipped
- When Case 2 is matched
- Statement of 2 will run
- Statements 1, 3, 4, and 5 are skipped
- When Case 3 is matched
- Statement of 3 will run
- Statements 1, 2, 4, and 5 are skipped
- When Case 4 is matched
- Statement of 4 will run
- Statements 1, 2, 3, and 5 are skipped
- When no case is matched
- Statement of 5 will run as this is the default case
- Statements 1, 2, 3, and 4 are skipped
Write a program to return weekday from its integer values
We want to return the weekday value in human-readable format from its corresponding integral representation.
- When the integer value is 1 then return Sunday
- When the integer value is 2 then return Monday
- When the integer value is 3 then return Tuesday
- When the integer value is 4 then return Wednesday
- When the integer value is 5 then return Thursday
- When the integer value is 6 then return Friday
- When the integer value is 7 then return Saturday
- When another value is there then print Invalid Value
package main
import "fmt"
func main() {
var day int = 3
switch day {
case 1:
fmt.Println("Sunday")
case 2:
fmt.Println("Monday")
case 3:
fmt.Println("Tuesday")
case 4:
fmt.Println("Wednesday")
case 5:
fmt.Println("Thursday")
case 6:
fmt.Println("Friday")
case 7:
fmt.Println("Saturday")
default:
fmt.Println("Invalid Value")
}
}
When the day value is 3 then the output is Tuesday
➜ go run main.go
Tuesday
You can change the day variable to 6 and rerun the program
➜ go run main.go
Friday
You can change the day variable to 100 and rerun the program. This time you will get the output value as an Invalid Value because the default case is executed
➜ go run main.go
Invalid Value