A variable in Golang is a storage location with a specific type and associated value.
- Declare a variable In Golang
- Initialize a variable in Golang
- Declare and Initialize a variable at the same time
- Rules of naming Golang Variable
- Scope of Variable
- Initialize and Declare multiple variables at once
- Program to convert from Fahrenheit to Celsius
- References
Declare a variable In Golang
The var keyword of Golang helps to create or declare a variable. There are three parts to it
- use the var keyword
- give a name to the variable
- assign the data type either integer or string etc
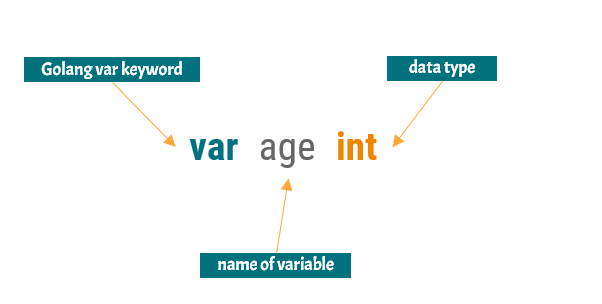
When you declare a variable then Golang assigns a default value to it based on the data type of the string
- The default value of an integer is 0
- The default value for a string in an empty string “”
- The default value for float is 0
var age int
Initialize a variable in Golang
Assigning a value to the variable is called an initialization. You can use the equal sign to assign the value based on the variable’s data type. In the below code, we are declaring the age variable as 10
var age int = 10
Declare and Initialize a variable at the same time
Golang provides a shorthand representation to declare and initialize the variable simultaneously. You can use the := symbol for this.
The type is not necessary because the Go compiler can infer the type based on the literal value you assign the variable
// this declares and initializes the variable at the same time
age := 10
Rules of naming Golang Variable
- The name must start with a letter
- It may contain underscore, letters, and numbers
- Go Compiler doesn’t care about the name of your variable but it should clearly describe the purpose of your variable
Scope of Variable
- The range of places where the variable can be used is called the scope of the variable.
- Go is lexically scoped using blocks.
- This means that the variable exists within the nearest curly braces ({}) or block including any nested curly blocks but not outside the block.
let’s create a main function define a variable and try to print it
package main
import "fmt"
func main() {
var name string = "Code with Raj Ranjan"
fmt.Println(name)
}
➜ go run main.go
Code with Raj Ranjan
- Output Analysis
- The name variable scope is within the nearest curly braces so if we try to print it within the curly braces then the variable is available and you can print it.
Create another function that prints the name variable
package main
import "fmt"
func main() {
var name string = "Code with Raj Ranjan"
fmt.Println(name)
// calling the new function
display()
}
// create new display function which tries to access the name variable
func display() {
fmt.Println(name)
}
➜ go run main.go
# command-line-arguments
./main.go:13:14: undefined: name
- Code Analysis
- we created a new function display which tries to print the name variable
- Output Analysis
- The Program throws an error as undefined: name
- As the name variable is not present in the scope of the display function it is unable to locate the name variable
Now move the name variable from the main function and put it at the top level
package main
import "fmt"
// define the name variable at top level block
var name string = "Code with Raj Ranjan"
func main() {
fmt.Println(name)
display()
}
func display() {
fmt.Println(name)
}
➜ go run main.go
Code with Raj Ranjan
Code with Raj Ranjan
- Code Analysis
- we moved the name variable outside the main function so the scope of the name variable is in full block of code
- Both the main function and display function can access it
- Output Analysis
- The program prints the text two times as both the main function and display function have scope access to the name variable
Initialize and Declare multiple variables at once
You can use a shorthand to initialize and declare multiple variables at once. You need to use the var keyword followed by parenthesis with each variable on its own line
Define and print three integers at once
package main
import "fmt"
func main() {
// define three integers at one time
var (
age = 10
count = 100
frequency = 50
)
fmt.Println(age)
fmt.Println(count)
fmt.Println(frequency)
}
➜ go run main.go
10
100
50
Define and print three strings at one time
package main
import "fmt"
func main() {
var (
name = "Code with Raj Ranjan"
country = "India"
city = "Bengaluru"
)
fmt.Println(name)
fmt.Println(country)
fmt.Println(city)
}
➜ go run main.go
Code with Raj Ranjan
India
Bengaluru
Program to convert from Fahrenheit to Celsius
The formula to convert from Fahrenheit to Celsius is
Celsius = (fahrenheit -32)* (5/9)
Let’s write a program for this
package main
import "fmt"
func main() {
// create a variable to store the fahrenheit temperature
var fahrenheit float64 = 132
// convert fahrenheit to celsius
celsius := (fahrenheit - 32) * 5 / 9
fmt.Println(celsius)
}
- Code Analysis
- we created a float variable fahrenheit
- Then calculated the Celsius values and stored it in the Celsius variable
- Then finally printed it