The variadic function in Golang supports accepting multiple input parameters with just one variable name. This is a special form available for the last parameter in any Golang function. The last argument of the function is preceded by three dots … called ellipsis
Singature of Variadic function in Golang
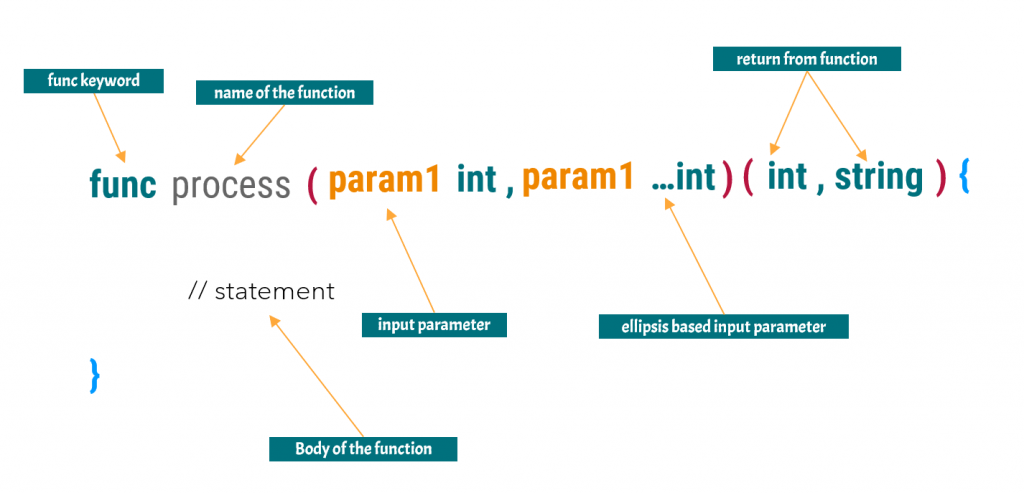
A variadic function has five parts
- func keyword of Golang which tells the compiler, we are going to define a function
- Name of the function: Here process is the name of the function
- You can give any name to your function.
- Providing meaningful names to the function increases the code readability
- Input Parameters
- These are the inputs given to a function
- There is no restriction on the number of input parameter
- It can be zero
- It can be one or more than one
- When you define the input parameter then you need to give two things
- Name of the input parameter as it will be used with this name inside the function body
- The data type of the input parameter
- When you define a variadic function then the last parameter data type is preceded with three dots … called ellipsis
- Return Values
- This defines what things can be returned from the function
- There is no restriction on the number of return values
- It can be zero
- It can be one or more than one
- You need to define the data type of the return values
- Body of the function
- These are the actual business logic statements that process input parameters and then return the values.
- The last parameter of the variadic function will become an array inside the body of the function and you can loop over it to get the value of each parameter.
Write a function that adds one or more numbers
Let’s create a function that adds one or more numbers using a variadic function. We are saying that the number of parameters is not fixed so it can be either one or ten or 100. For handling this case we will define the last input parameter in the ellipsis format
func add(numbers ...int) (int) {
// some business logic
}
Also, the ellipsis-based parameter is like an array inside the function body. we can loop over it and find the sum. The complete function is written below
func add(numbers ...int) int {
sum := 0
// input paramter is available as an array
for _, number := range numbers {
sum += number
}
return sum
}